Today let us learn about novalidate and formnovalidate attributes of HTML5 form.
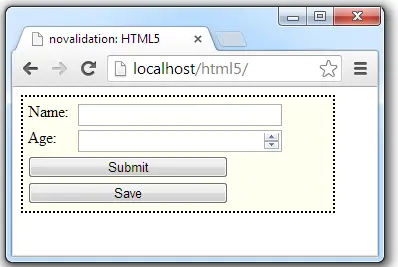
In this video tutorial I’m taking 2 input fields and making one input field as required. Also take 2 submit buttons, with one validating the user entered data and the other one bypassing the validation rules set.
HTML file: formnovalidate
index.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 | < !DOCTYPE html> <html> <head> <title>novalidation: HTML5</title> <link href="myStyle.css" rel="stylesheet"/> </head> <body> <form> <label for="name">Name: </label> <input type="text" name="name" required/><br /> <label for="age">Age: </label> <input type="number" name="age"/><br /> <input type="submit" value="Submit"/> <input type="submit" value="Save" formnovalidate/> </form> </body> </html> |
Here we are using formnovalidate attribute on submit button which has a value of Save. This button bypasses the validation rules.
HTML file: novalidate
index.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 | < !DOCTYPE html> <html> <head> <title>novalidation: HTML5</title> <link href="myStyle.css" rel="stylesheet"/> </head> <body> <form novalidate> <label for="name">Name: </label> <input type="text" name="name" required/><br /> <label for="age">Age: </label> <input type="number" name="age"/><br /> <input type="submit" value="Submit"/> </form> </body> </html> |
Here I’ve added novalidate attribute directly to the form tag, which bypasses any validation rules written to it or to it’s child elements/tags.
CSS file associated with this is same as present at pattern and title Attribute of Form Field: HTML5
novalidate and formnovalidate attributes of HTML5 form
[youtube https://www.youtube.com/watch?v=BbU2KndW7Ho]
Why would we ever need novalidation for forms ?
Sometimes we enter a lengthy form and submit, but due to some validation mistakes all our entries gets erased, and the user may not have the patience to re-enter everything from beginning. He may skip it altogether. To avoid that, you can build an interface wherein user can save her data first and then can submit it after reviewing it. In such a situation we can make use of a temporary database table to store user entered information and display for review with appropriate messages to correct the wrong inputs – user can correct them and Submit.