In this video tutorial we shall briefly look at Express web framework for Node.js
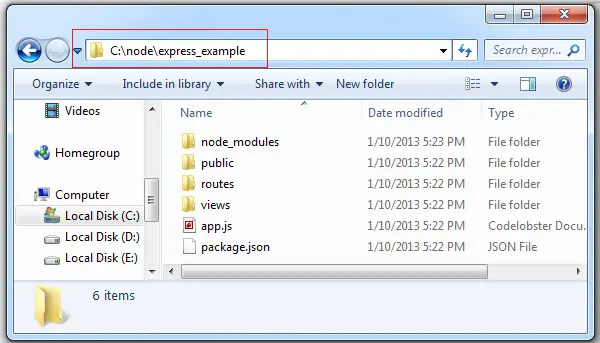
With today’s tutorial we will be discussing the basics of Express and we’ll also be running a small example application built with Express, also we shall have a first look at Jade Template Engine.
REPL
global installation of Express Framework
C:\>npm install -g express
This installs the express globally, so that you can access it from anywhere in the system.
Also note that, you’ll need internet connection to download and install these packages.
REPL
creating express example application
C:\>cd node C:\node>express express_example
This creates a folder and some recommended folder structure. If you’re a total beginner to Express Framework, then it’s better to stick on with these folder structure.
Now open the package.json present inside express_example folder
JSON File
package.json
{ "name": "application-name", "version": "0.0.1", "private": true, "scripts": { "start": "node app.js" }, "dependencies": { "express": "3.4.0", "jade": "*" } }
It looks as shown above. It has 2 dependencies: express and jade. Install these dependencies from your command prompt / console.
REPL
installing dependencies
C:\>cd node C:\node>cd express_example C:\node\express_example>nmp install
This would install both express and jade, and another folder called node_modules gets created inside express_example folder.
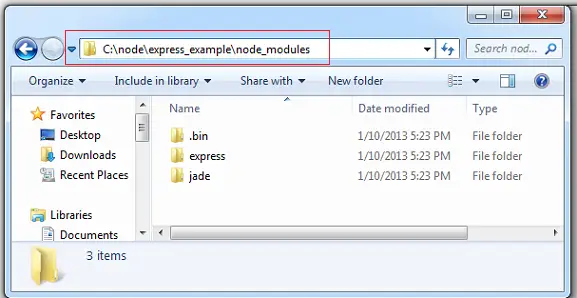
Video Tutorials: Getting Started With Express Web Framework: Node.js
[youtube https://www.youtube.com/watch?v=CagrpUlQtdQ]
Jade File
Jade to HTML
extends layout block content h1= title p Welcome to #{title}
This jade file is present inside view folder.
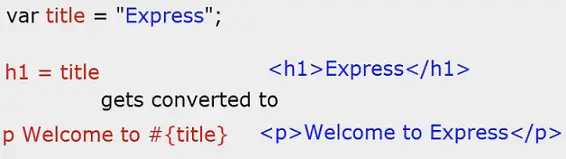
More about Jade Template Engine in coming videos ..
Note:
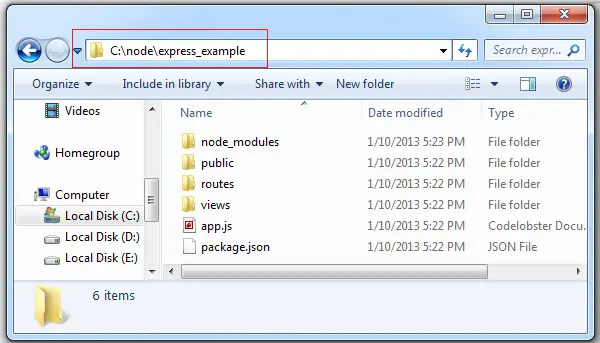
Inside node_modules folder we have dependency modules.
Inside public folder javascript files, css files, images etc are present. Logical part of the application will be kept separate for security reasons.
Inside routes, routing configuration is present.
Inside views, the presentation part of the application is present. If Template Engine is used, those files will be present in this folder. Example: .jade files
app.js Starting point of execution.
package.json Contains app name, version, author, dependency module names etc.
Info: There are many popular websites built upon Express Framework, one that you might know is, MySpace!