Today lets build a dynamic suggested list input field using HTML5, jQuery, PHP and MySQL.
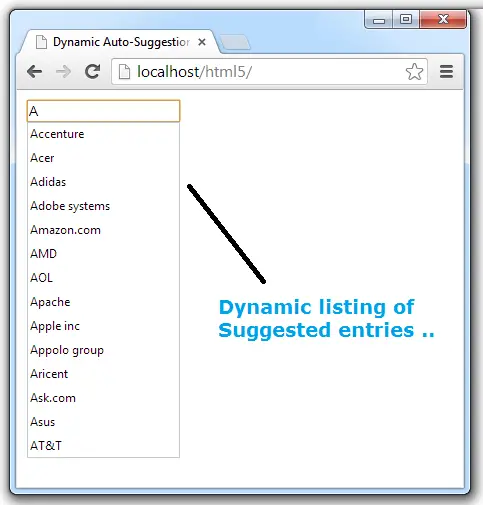
We have 55 company names in our database table – and the import file(.sql) can be downloaded at the end of this tutorial page. By using jQuery we pass user input to suggest.php file and the result is embedded into index.html file.
Related read: Suggested Entries Using datalist Element: HTML5
HTML file
index.html
<!DOCTYPE HTML> <html> <head> <title>Dynamic Auto-Suggestion using datalist element: HTML5</title> <meta charset="utf-8"/> <script src="jQuery.js"></script> <script src="myScript.js"></script> </head> <body> <form> <input type="text" list="myCompanies" name="company" id="suggest" /> <datalist id="myCompanies"> </datalist> </form> </body> </html>
Here we have included jQuery library file before our script myScript.js
We also have an input field of type text, with an id = suggest, name = company and list = myCompanies. And a datalist element is linked/associated with input field, by matching it’s id with the name of input field’s list attribute. The value for option tag will be filled by jQuery, using the results output by suggest.php file.
PHP file
suggest.php
< ?php $db = mysqli_connect('localhost', 'root', '', 'search'); $company = $_GET['company']; $sql = "SELECT name FROM table1 WHERE name like '$company%' ORDER BY name"; $res = $db->query($sql); if(!$res) echo mysqli_error($db); else while( $row = $res->fetch_object() ) echo "<option value="".$row->name."">"; ?>
Here we connect php file to database(search). Get the keyword entered by the user in the input field and form a sql query. By using query() method, we process it and finally format it the way we need it i.e., we wrap the company names inside option tag’s value attribute.
jQuery file
myScript.js
$(document).ready(function(){ $("#suggest").keyup(function(){ $.get("suggest.php", {company: $(this).val()}, function(data){ $("datalist").empty(); $("datalist").html(data); }); }); });
For every keyup event on the input field we trigger jQuery method $.get() and pass the user input keys to suggest.php file and the result is collected back by the callback function and the result set is stored in a variable called data.
Now we first make sure to clear out previous data present inside datalist element i.e., we clear the previous results by removing any option tags present inside datalist element. Now we fill the datalist element with the new data/result output from suggest.php file.
Since we’re using jQuery here, page does not reload and the suggested entries looks real and spontaneous.
Here is the list of company names I’ve taken for this tutorial
3M
7-Eleven
Accenture
Acer
Adidas
Adobe systems
Amazon.com
AMD
AOL
Apache
Apple inc
Appolo group
Aricent
Ask.com
Asus
AT&T
Bank of America
BBC
BE Aerospace
BMC Software
Boeing
Bosch Brewing Company
Boston Acoustic
CA Technologies
Citrix Systems
Cognizant Technolog
Cognizant Technology Solutions
Convergys
Dell
Delphi
DHL
Divx Inc
eBay
EMC Corporation
Exelon
Facebook
Ford Motor Company
Fox Entertainment Group
GCI
GoDaddy
Goodrich Corporation
Google
Hawaiian Airlines
Hewlett-Packard
Honeywell
IBM
Intel
Johnson & Johnson
KFC
McDonalds
Microsoft
Motorola
Nvidia
Red Hat
Yahoo!
Video Tutorials: Dynamic Suggested List: HTML5 + jQuery + PHP + MySQL
[youtube https://www.youtube.com/watch?v=YqMtE8UO-xw]
Note: For any older browsers which do not support datalist element or list attribute, it will simply fall back to text type input field and it doesn’t produce any error.