Lets see how require attribute of HTML5 works.
require attribute forces the user to enter some value. But it does not validate the user input.
Example: It makes sure if the email field has some value in it but doesn’t check if the user has entered valid email address or not. It only makes sure, the required field isn’t left empty.
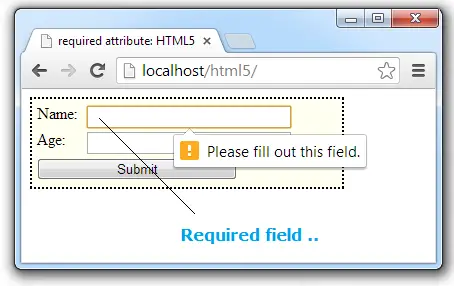
HTML file
index.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 | < !DOCTYPE html> <html> <head> <title>required attribute: HTML5</title> <link href="myStyle.css" rel="stylesheet"/> </head> <body> <form> <label for="name">Name: </label> <input type="text" name="name" required/><br /> <label for="age">Age: </label> <input type="text" name="age"/><br /> <input type="submit"/> </form> </body> </html> |
Here we have 2 input fields. First input field has been made required, by using require attribute.
CSS file associated with this is same as present at pattern and title Attribute of Form Field: HTML5
require attribute: HTML5
[youtube https://www.youtube.com/watch?v=yEVyYQjMaMU]
Note:
Server side validation is also equally important. Because someone might directly pass the value via the script present in the action field of form tag – if form uses GET method to pass user data.
Also note that, IE(Internet Explorer) doesn’t support require attribute, so you can make use of Javascript or jQuery to make sure IE users are taken care of.
Related Read: Registration Form Validation: PHP + jQuery + AJAX