After learning to connect our Node.js application to MongoDB, lets see how we could insert data into our database collection.
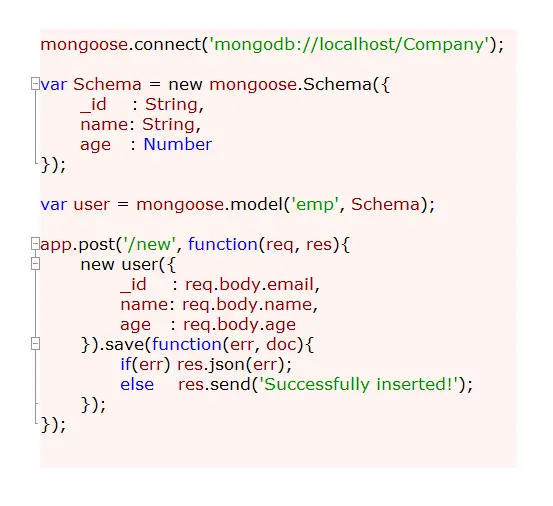
Note:
Save() is a higher lever method. It checks to see if the object we’re inserting is already present in our collection, by checking the _id value we’re inserting. _id field(which is a primary key in MongoDB document)
– If there is no matching _id field in a document, it’ll call insert method and insert the data. And if the data being entered has no _id value, insert() method will create _id value for us, and insert the data into the collection.
– If _id matches to any document in our collection, it’ll use update() instead to update the previously present data with the new data entered by the user.
Form To Facilitate User To Enter Her Details ..or Registration Form
public/index.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | < !DOCTYPE html> <html> <head> <title>Registration Form</title> </head> <body> <form action="/new" method="POST"> <label for="email">Email: </label> <input type="email" name="email" /><br /> <label for="name">Name: </label> <input type="text" name="name" /><br /> <label for="age">Age: </label> <input type="number" name="age" /><br /> <input type="submit"/> </form> </body> </html> |
Here we have 3 input fields(for email, name and age) and a submit button. Since we’re posting these data to /new (in the action field of the form), we need to create /new route.
Route To Process User Entries
app.js
1 2 3 4 5 6 7 8 9 10 | app.post('/new', function(req, res){ new user({ _id : req.body.email, name: req.body.name, age : req.body.age }).save(function(err, doc){ if(err) res.json(err); else res.send('Successfully inserted!'); }); }); |
By using bodyParser() middleware, we get the data from the form, parse it and assign the values to corresponding keys, by creating a new user.
Note: user is a model object in our example. We also chain save method with new user object. Save() method takes a callback method as argument. The callback method takes 2 arguments – error and document. We check, if there are any errors while inserting the data, if so, we output the error object on to the screen. If the insertion was successful, we display “Successfully inserted!” message.
Inserting data To MongoDB: Node.js
[youtube https://www.youtube.com/watch?v=uZqwHfNIf8M]
Related Read:
number Input Type: HTML5
Create and Insert Documents: MongoDB
MongoDB Tutorial List
Full Code
app.js
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 | var express = require('express'); var http = require('http'); var path = require('path'); var mongoose = require('mongoose'); var app = express(); // all environments app.set('port', process.env.PORT || 3000); app.set('views', __dirname + '/views'); app.set('view engine', 'jade'); app.use(express.bodyParser()); app.use(express.methodOverride()); app.use(app.router); app.use(express.static(path.join(__dirname, 'public'))); mongoose.connect('mongodb://localhost/Company'); var Schema = new mongoose.Schema({ _id : String, name: String, age : Number }); var user = mongoose.model('emp', Schema); app.post('/new', function(req, res){ new user({ _id : req.body.email, name: req.body.name, age : req.body.age }).save(function(err, doc){ if(err) res.json(err); else res.send('Successfully inserted!'); }); }); var server = http.createServer(app).listen(app.get('port'), function(){ console.log('Express server listening on port ' + app.get('port')); }); |
Here we’ve shown the configurations, middleware, mongoose connection, schema, model object, routes and out application server.