This video tutorial illustrates loading data to mongoDB server from an external JavaScript file ( Writing script for MongoDB shall )
Here, we write a simple JavaScript file and using command prompt we load the contents of JavaScript file into new Database.
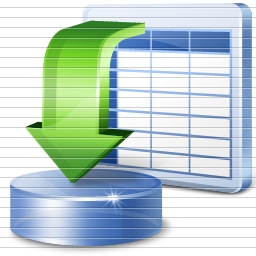
JavaScript file
load.js – in path: C:/temp/load.js
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | db.person.insert({ name:'Satish', age:25, skills:['nodejs', 'mongoDB', 'HTML5'] }); db.person.insert({ name:'Kiran', age:27, skills:['PHP', 'mySQL', 'HTML5'] }); db.person.insert({ name:'Sunitha', age:24, skills:['html', 'ASP'] }); |
Here we’re inserting some data/record/documents into the collection person.
Database’s Before running the script
1 2 3 4 5 6 7 8 9 10 11 12 13 | C:\>cd mongodb C:\mongodb>cd bin C:\mongodb\bin>mongo MongoDB shell version: 2.4.3 connecting to: test > show dbs admin 0.203125GB company 0.203125GB local 0.078125GB > exit bye |
Before running the script, we have only 3 databases.
Running the script
1 2 3 | C:\mongodb\bin>mongo 127.0.0.1/satish C:/temp/load.js MongoDB shell version: 2.4.3 connecting to: 127.0.0.1/satish |
Here, mongo is the JavaScript shall.
127.0.0.1 is nothing but our localhost.
satish is the new database we are creating.
C:/temp/load.js is the path of load.js file.
We’re loading the contents of load.js file into new database satish.
Database’s After running the script
1 2 3 4 5 6 7 8 9 10 11 12 13 | C:\mongodb\bin>mongo MongoDB shell version: 2.4.3 connecting to: test > show dbs admin 0.203125GB company 0.203125GB local 0.078125GB satish 0.203125GB > use satish switched to db satish > show collections person system.indexes |
New database satish has been added and it has person collection, which we loaded from the JavaScript file.
Documents/records In person collection
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 | > db.person.find().forEach(printjson) { "_id" : ObjectId("518b62443cbad352108c321b"), "name" : "Satish", "age" : 25, "skills" : [ "nodejs", "mongoDB", "HTML5" ] } { "_id" : ObjectId("518b62443cbad352108c321c"), "name" : "Kiran", "age" : 27, "skills" : [ "PHP", "mySQL", "HTML5" ] } { "_id" : ObjectId("518b62443cbad352108c321d"), "name" : "Sunitha", "age" : 24, "skills" : [ "html", "ASP" ] } |
Using find() method we display all its contents.
This way we could load application data from an external JavaScript file.
Writing script for MongoDB shall
[youtube https://www.youtube.com/watch?v=ygK2zE1-k0w]
Note:
This method will be handy while migrating our application from one mongoDB server to another mongoDB server.
There is import/export options in mongoDB, but this method is also helpful if we have some custom data to be inserted. nontheless a useful tool to have.