Today lets learn some very important input types of HTML5:
datetime
datetime-local
date
time
week
month
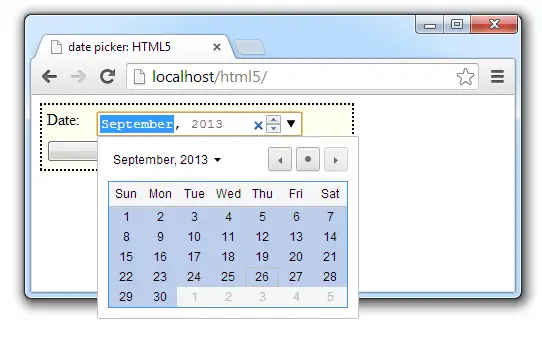
datetime input type is supported by Opera browser, but as of now(2013), Google Chrome doesn’t support it. All other input date time related input types are supported by Google Chrome.
HTML file: month input type
index.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | < !DOCTYPE html> <html> <head> <title>date picker: HTML5</title> <link href="myStyle.css" rel="stylesheet" /> </head> <body> <form> <label for="dt">Date: </label> <input name="dt" type="month" min="2013-01" max="2013-09"/> <input type="submit"/> </form> </body> </html> |
Here we have a month type input field which has a minimum allowed month as 2013-01 and a maximum allowed month as 2013-09.
HTML file: date input type
index.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | < !DOCTYPE html> <html> <head> <title>date picker: HTML5</title> <link href="myStyle.css" rel="stylesheet" /> </head> <body> <form> <label for="dt">Date: </label> <input name="dt" type="date"/> <input type="submit"/> </form> </body> </html> |
Here we have a date input type, which shows a date picker in the UI.
HTML file: time input type
index.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | < !DOCTYPE html> <html> <head> <title>date picker: HTML5</title> <link href="myStyle.css" rel="stylesheet" /> </head> <body> <form> <label for="dt">Date: </label> <input name="dt" type="time"/> <input type="submit"/> </form> </body> </html> |
Here we have a time input type, we could even specify the fractional seconds. Look for the syntax at the end of this post.
HTML file: datetime input type
index.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | < !DOCTYPE html> <html> <head> <title>date picker: HTML5</title> <link href="myStyle.css" rel="stylesheet" /> </head> <body> <form> <label for="dt">Date: </label> <input name="dt" type="datetime"/> <input type="submit"/> </form> </body> </html> |
datetime input type which signifies Universal Time Convention(UTC).
HTML file: datetime-local input type
index.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | < !DOCTYPE html> <html> <head> <title>date picker: HTML5</title> <link href="myStyle.css" rel="stylesheet" /> </head> <body> <form> <label for="dt">Date: </label> <input name="dt" type="datetime-local"/> <input type="submit"/> </form> </body> </html> |
Here we have a datetime-local input type, which takes the input based on the local time zone of the user’s machine.
HTML file: week input type
index.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | < !DOCTYPE html> <html> <head> <title>date picker: HTML5</title> <link href="myStyle.css" rel="stylesheet" /> </head> <body> <form> <label for="dt">Date: </label> <input name="dt" type="week"/> <input type="submit"/> </form> </body> </html> |
week input type gives date picker UI and allows user to select the entire date.
Form Input Type – date time: HTML5
[youtube https://www.youtube.com/watch?v=VKRuSryBaNk]
Related Read: Format Date and Insert Into Database Table: PHP
Note:
We can specify value, min, max and step attributes for all these input types. And the format for it is as shown below:
date YYYY-MM-DD default step 60 seconds
time HH:MM:SS.FF default step 1 Day (SS.FF means seconds and fractional seconds)
datetime YYYY-MM-DDTHH:MM:SS.FFZ default step 60 seconds (T and Z means the TimeZone, these alphabets/characters must be literally present in the format)
datetime-local YYYY-MM-DDTHH:MM:SS.FF default step 60 seconds (albhabet/character/letter T must be literally present in the format)
week YYYY-WNN default step of 1 week (letter W means the week, this alphabet/characters must be literally present in the format)
month YYYY-MM default step of 1 month