Write a function to compute the distance between two points and use it to develop another function that will compute the area of the triangle whose vertices are A(x1, y1), B(x2, y2), and C(x3, y3). Use these functions to develop a function which returns a value 1 if the point (x, y) lies inside the triangle ABC, otherwise returns a value 0.
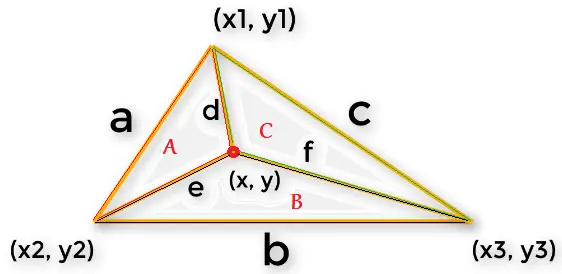
Analyze Above Problem Statement
1. We need to define a function which calculates the distance between two points.
For Example: distance(x1, y1, x2, y2); This function takes values of 2 points (x1, y1) and (x2, y2) and returns the distance between these 2 points.
2. User will enter values for 3 points, which are 3 edges/points of the triangle: (x1, y1), (x2, y2) and (x3, y3). We need to make use of a function(check Step 1) to calculate the distance between them. Once we have the distances, which are nothing but 3 sides of the triangle, we can use it to find area of that triangle using Heron’s or Hero’s Formula.
We call the distance between points (x1, y1) and (x2, y2) as a.
We call the distance between points (x2, y2) and (x3, y3) as b.
We call the distance between points (x3, y3) and (x1, y1) as c.
Using 3 sides of the triangle and Heron’s formula we calculate the area of triangle and store it inside variable area.
Hint: Check the image above for all these points we’ve explained so far. It has all the markings, which will help you visualize the concept.
3. Next, user will enter values for a point(x, y) on the graph. We need to make use of distance function(see step 1) and area of triangle function(see step 2) to find the area of 3 triangles formed by connecting (x1, y1) and (x, y), (x2, y2) and (x, y), (x3, y3) and (x, y);
We store the area of these 3 triangles inside variables A, B and C.
4. Write a function which returns 1 if the point lies within the triangle and returns 0 if the point lies outside the triangle.
If area of triangle represented by points (x1, y1), (x2, y2) and (x3, y3) is equal to the sum of the 3 triangles formed by connecting these 3 points to (x, y), then the points lie inside the triangle else the point is outside the triangle.
i.e., area_of_main_triangle == A + B + C; then the point lies inside the main triangle else it lies outside the main triangle.
Hint: Check the image above once again and write it down on a piece of paper, so that you clearly understand all the markings.
Note: This C program is comparatively bigger, but most part of its logic is already covered in other videos. Nothing complicated here. Its very simple, easy and straightforward program. Just don’t get stressed by looking at the length of this program. Learn the code(and the logic) step by step. Consume this or understand this program bit by bit and in no time you’ll know the whole thing!
Related Read:
C Program To Calculate Distance Between Two Points
C Program To Calculate Area of a Triangle using Pointers
Video Tutorial: C Program To Find If a Point Lies Inside Triangle or Not
[youtube https://www.youtube.com/watch?v=ntjM9YZP0qk]
Source Code: C Program To Find If a Point Lies Inside Triangle or Not
#include<stdio.h>
#include<math.h>
void calc(float x1, float y1, float x2, float y2, float x3, float y3,
float x, float y, int *flag, float *area);
int position(float area, float A, float B, float C);
float distance(float x1, float y1, float x2, float y2);
float calc_area(float a, float b, float c);
int main()
{
float x1, y1, x2, y2, x3, y3, x, y;
int flag = 0;
float area = 0;
printf("Enter value for(x1, y1)\n");
scanf("%f%f", &x1, &y1);
printf("Enter value for(x2, y2)\n");
scanf("%f%f", &x2, &y2);
printf("Enter value for(x3, y3)\n");
scanf("%f%f", &x3, &y3);
printf("Enter point(x, y) to check if it lies inside the Triangle\n");
scanf("%f%f", &x, &y);
calc(x1, y1, x2, y2, x3, y3, x, y, &flag, &area);
printf("\nArea of Triangle = %f\n", area);
if(flag) printf("Point (%0.1f, %0.1f) lies within the Triangle\n", x, y);
else printf("Point (%0.1f, %0.1f) lies outside the Triangle\n", x, y);
return 0;
}
void calc(float x1, float y1, float x2, float y2, float x3, float y3,
float x, float y, int *flag, float *area)
{
float A, B, C, a, b, c, d, e, f;
a = distance(x1, y1, x2, y2);
b = distance(x2, y2, x3, y3);
c = distance(x3, y3, x1, y1);
*area = calc_area(a, b, c);
d = distance(x1, y1, x, y);
e = distance(x2, y2, x, y);
f = distance(x3, y3, x, y);
A = calc_area(d, a, e);
B = calc_area(e, b, f);
C = calc_area(f, c, d);
*flag = position(*area, A, B, C);
}
float distance(float x1, float y1, float x2, float y2)
{
return( sqrt( pow((x2 - x1), 2) + pow((y2 - y1), 2) ) );
}
float calc_area(float a, float b, float c)
{
float S;
S = (a + b + c) / 2.0;
return( sqrt(S * (S - a) * (S - b) * (S - c) ) );
}
int position(float area, float A, float B, float C)
{
float res = area - (A + B + C);
if(res < 0)
{
res *= -1;
}
if(res == 0 || res < 0.001 )
{
return(1);
}
else
{
return(0);
}
}
Output 1:
Enter value for(x1, y1)
0 0
Enter value for(x2, y2)
10 0
Enter value for(x3, y3)
10 20
Enter point(x, y) to check if it lies inside the Triangle
5 2
Area of Triangle = 100.000000
Point (5.0, 2.0) lies within the Triangle
Output 2:
Enter value for(x1, y1)
0 0
Enter value for(x2, y2)
10 0
Enter value for(x3, y3)
10 20
Enter point(x, y) to check if it lies inside the Triangle
10 20.1
Area of Triangle = 100.000000
Point (10.0, 20.1) lies outside the Triangle
Output 3:
Enter value for(x1, y1)
0 0
Enter value for(x2, y2)
20 0
Enter value for(x3, y3)
10 30
Enter point(x, y) to check if it lies inside the Triangle
10 15
Area of Triangle = 300.000000
Point (10.0, 15.0) lies within the Triangle
Logic To Find If a Point Lies Inside Triangle or Not
We ask the user to enter values for 3 edges or points of the triangle. We call it (x1, y1), (x2, y2) and (x3, y3). We also ask the user to enter a point, to check if it lies inside or outside the Triangle. We store the value of point inside (x, y).
We pass the values of (x1, y1), (x2, y2), (x3, y3), (x, y) and the address of variables flag and area to a function called calc().
calc(x1, y1, x2, y2, x3, y3, x, y, &flag, &area);
Inside calc() function
Inside calc() function we call another function to find distance between user entered points (x1, y1), (x2, y2), (x3, y3) and (x, y). We pass values of 2 points to the function distance() to get back the value or the distance between these two points.
a = distance(x1, y1, x2, y2);
Similarly, we find the distance between points (x2, y2) and (x3, y3), (x3, y3) and (x1, y1). This gives us lengths of all 3 sides of the triangle.
a = distance(x1, y1, x2, y2);
b = distance(x2, y2, x3, y3);
c = distance(x3, y3, x1, y1);
Inside distance() function
We use a simple formula to calculate distance between 2 points:
distance = sqrt( (x2 – x1)*(x2 – x1) + (y2 – y1)*(y2 – y1) );
C Program To Calculate Distance Between Two Points
Inside calc_area() function
When we know the values of all 3 sides of a triangle, we can easily calculate its area using Heron’s or Hero’s formula.
sqrt(S * (S – a) * (S – b) * (S – c) );
where a, b and c are sides of the triangle.
and S is semi-perimeter of the Triangle.
S is calculate using below formula:
S = (a + b + c) / 2.0;
C Program To Calculate Area of a Triangle using Pointers
Inside calc() function
Now we calculate the distance between the user entered point(x, y) and with all 3 points of the triangle (x1, y1), (x2, y2) and (x3, y3). And store the values inside variables d, e, f. We make use of the function distance() here to get the distance between the points.
Inside calc_area() function
Again we reuse the calc_area() function to calculate the area’s of 3 triangles formed by connecting (x1, y1), (x2, y2), (x3, y3) with (x, y).
Inside position() function
Now we have area of the entire triangle(with sides a, b, c). And area of 3 more triangles present inside the main triangle – according to our assumption, as per above image. It need not be like that. We store these 3 areas in variables A, B and C.
If area == A + B + C, then the point (x, y) lies inside the triangle orelse the point(x, y) lies outside the triangle.
Very Important Note:
Since variables *area, A, B, C and all the points on the graph are of type float. So the final result for *area == A + B + C will have a very small difference. i.e., up to 0.001
So whenever we check for if(*area == A + B + C) it returns false or 0. So we use different logic. If the point lies inside the triangle then A + B + C will be equal to the area of main triangle. So we subtract the value of main triangle with all the 3 triangles present inside, so the result should be either 0 or with a small difference less than 0.001.
So the difference can be positive or negative, so we multiple the result by -1 in case the result is less than 0. After that we check for the condition if res is equal to 0 or res is less than 0.001, then we return 1, indicating that the point lies inside the triangle. If there is a difference bigger than 0.001 then we return 0, indicating that the point (x, y) lies outside the triangle.
Source Code Which Matches Exactly To The Problem Statement
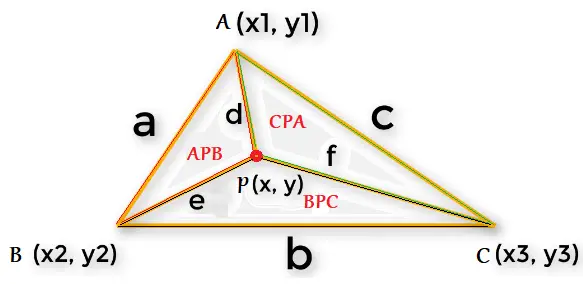
We’ve changed the variable names to match the problem statement. Rest everything is same – the logic and working etc. Only 4 variable names have been changed to match the problem statement.
Source Code: C Program To Find If a Point Lies Inside Triangle or Not
#include<stdio.h>
#include<math.h>
void calc(float x1, float y1, float x2, float y2, float x3, float y3,
float x, float y, int *flag, float *ABC);
int position(float ABC, float APB, float BPC, float CPA);
float distance(float x1, float y1, float x2, float y2);
float calc_area(float a, float b, float c);
int main()
{
float x1, y1, x2, y2, x3, y3, x, y;
int flag = 0;
float ABC = 0;
printf("Enter value for(x1, y1)\n");
scanf("%f%f", &x1, &y1);
printf("Enter value for(x2, y2)\n");
scanf("%f%f", &x2, &y2);
printf("Enter value for(x3, y3)\n");
scanf("%f%f", &x3, &y3);
printf("Enter point(x, y) to check if it lies inside the Triangle\n");
scanf("%f%f", &x, &y);
calc(x1, y1, x2, y2, x3, y3, x, y, &flag, &ABC);
printf("\nArea of Triangle = %f\n", ABC);
if(flag) printf("Point (%0.1f, %0.1f) lies within the Triangle\n", x, y);
else printf("Point (%0.1f, %0.1f) lies outside the Triangle\n", x, y);
return 0;
}
void calc(float x1, float y1, float x2, float y2, float x3, float y3,
float x, float y, int *flag, float *ABC)
{
float APB, BPC, CPA, a, b, c, d, e, f;
a = distance(x1, y1, x2, y2);
b = distance(x2, y2, x3, y3);
c = distance(x3, y3, x1, y1);
*ABC = calc_area(a, b, c);
d = distance(x1, y1, x, y);
e = distance(x2, y2, x, y);
f = distance(x3, y3, x, y);
APB = calc_area(d, a, e);
BPC = calc_area(e, b, f);
CPA = calc_area(f, c, d);
*flag = position(*ABC, APB, BPC, CPA);
}
int position(float ABC, float APB, float BPC, float CPA)
{
float res = ABC - (APB + BPC + CPA);
if(res < 0)
{
res *= -1;
}
if(res == 0 || res < 0.001 )
{
return(1);
}
else
{
return(0);
}
}
float distance(float x1, float y1, float x2, float y2)
{
return( sqrt( pow((x2 - x1), 2) + pow((y2 - y1), 2) ) );
}
float calc_area(float a, float b, float c)
{
float S;
S = (a + b + c) / 2.0;
return( sqrt(S * (S - a) * (S - b) * (S - c) ) );
}
Output 1:
Enter value for(x1, y1)
0 0
Enter value for(x2, y2)
10 0
Enter value for(x3, y3)
10 20
Enter point(x, y) to check if it lies inside the Triangle
5 2
Area of Triangle = 100.000000
Point (5.0, 2.0) lies within the Triangle
Output 2:
Enter value for(x1, y1)
0 0
Enter value for(x2, y2)
10 0
Enter value for(x3, y3)
10 20
Enter point(x, y) to check if it lies inside the Triangle
10 20.1
Area of Triangle = 100.000000
Point (10.0, 20.1) lies outside the Triangle
Output 3:
Enter value for(x1, y1)
0 0
Enter value for(x2, y2)
20 0
Enter value for(x3, y3)
10 30
Enter point(x, y) to check if it lies inside the Triangle
10 15
Area of Triangle = 300.000000
Point (10.0, 15.0) lies within the Triangle
For list of all c programming interviews / viva question and answers visit: C Programming Interview / Viva Q&A List
For full C programming language free video tutorial list visit:C Programming: Beginner To Advance To Expert