Today lets learn how to use arcs and paths to draw circle.
Arcs are curves that are portions of a circle. A full circle is a 360 degree arc!
A Path is a collection of some lines(or shapes) that once defined can be either filled or stroked.
index.html and myStyle.css files are kept same as illustrated in previous video tutorial: Canvas State: HTML5
Syntax
arc(x, y, r, sA, eA, TF);
arc method takes 6 parameters. x and y are the x-axis and y-axis, which is the center of the circle.
i.e., The x and y parameters determine where the center of the circle will be located on your canvas.
r is the radius of the circle, in pixels.
i.e., the distance of the curve from the center of the circle.
sA is start angle of the circle.
eA is the end angle of the circle.
TF is the direction – which can take 2 values i.e., True or False.
True: negative degrees, counter-clockwise direction.
False: positive degrees, clockwise direction.
Javascript file
myScript.js
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 | window.onload = canvas; function canvas() { var myCanvas = document.getElementById("myCanvas"); if( myCanvas && myCanvas.getContext("2d") ) { var context = myCanvas.getContext("2d"); context.lineWidth= 6; context.beginPath(); var degree = 270; var radian = ( Math.PI / 180 ) * degree; context.arc(100, 100, 50, 0, radian, false); context.strokeStyle= "red"; context.fillStyle= "blue"; context.stroke(); context.fill(); context.closePath(); } } |
Degree to Radian
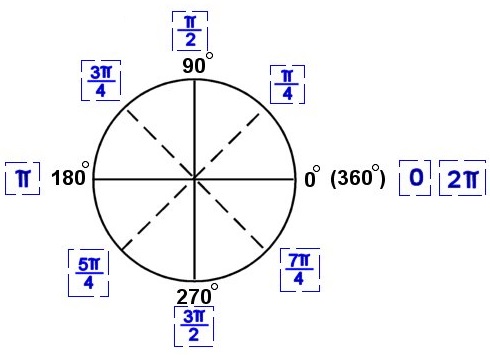
We convert degrees to radians using a simple mathematical formula:
Radians = (PI / 180 ) * degree;
Draw Arcs/Circle with Canvas: HTML5
[youtube https://www.youtube.com/watch?v=vE0bstctkp4]
Note:
1. By default, direction of drawing the arc is clockwise/positive degrees(i.e., the value of last parameter is false).
2. PI value is 180.
3. 2 * PI is 360!
4. arc method should be passed with radian values and not degrees.