Lets write a C program to draw / print / display a pyramid / triangle formed from uppercase / capital letter English Alphabets, using while loop.
Related Read:
Nested While Loop: C Program
C Program To Draw Pyramid of Stars, using While Loop
Expected Output for the Input
User Input:
Enter the number of rows for Pyramid
5
Output:
A
BCD
EFGHI
JKLMNOP
QRSTUVWXY
Pyramid With 20 Rows
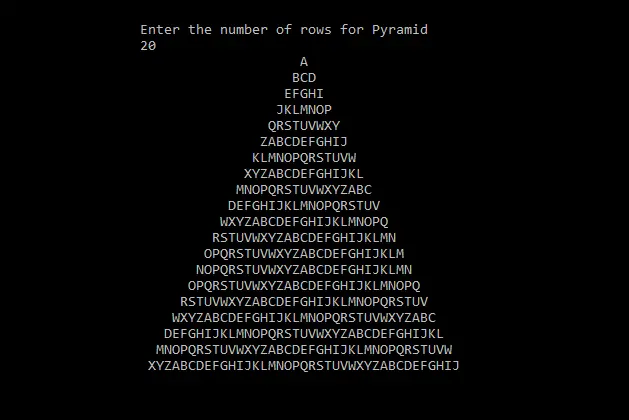
Video Tutorial: C Program To Draw Pyramid of Alphabets, using While Loop
[youtube https://www.youtube.com/watch?v=eDPYA_c73I4]
Logic To Draw Pyramid of Alphabets, using While Loop
We ask the user to enter the maximum number of rows for the pyramid and store it inside the address of variable num. We declare and initialize a variable count to 1(indicating first row of the pyramid).
In the outer while loop we check if count is less than or equal to num. For each iteration of the while loop we increment the value of count by 1. So the count value will have the row number i.e., for each iteration of the outer while loop we select row one by one (to print the alphabets).
Inside first inner while loop we print the adequate number of space for each row. Inside the second inner while loop we actually print the alphabets needed for each row.
At the end the result will be a pyramid with the number of rows as input by the user.
ASCII Value of Capital Letter English Alphabets
ASCII value of A is 65
ASCII value of B is 66
ASCII value of C is 67
ASCII value of D is 68
ASCII value of E is 69
ASCII value of F is 70
ASCII value of G is 71
ASCII value of H is 72
ASCII value of I is 73
ASCII value of J is 74
ASCII value of K is 75
ASCII value of L is 76
ASCII value of M is 77
ASCII value of N is 78
ASCII value of O is 79
ASCII value of P is 80
ASCII value of Q is 81
ASCII value of R is 82
ASCII value of S is 83
ASCII value of T is 84
ASCII value of U is 85
ASCII value of V is 86
ASCII value of W is 87
ASCII value of X is 88
ASCII value of Y is 89
ASCII value of Z is 90
Reference: C Program To Print All ASCII Characters and Code
Source Code: C Program To Draw Pyramid of Alphabets, using While Loop
#include < stdio.h >
int main()
{
int num, count = 1, i, alphabet = 65;
printf("Enter the number of rows for Pyramid\n");
scanf("%d", &num);
while(count <= num)
{
i = 0;
while(i <= (num-count))
{
printf(" ");
i++;
}
i = 0;
while(i < (2*count-1))
{
printf("%c", alphabet);
if(alphabet == 90)
{
alphabet = 64;
}
alphabet++;
i++;
}
printf("\n");
count++;
}
return 0;
}
Output
Enter the number of rows for Pyramid
14
A
BCD
EFGHI
JKLMNOP
QRSTUVWXY
ZABCDEFGHIJ
KLMNOPQRSTUVW
XYZABCDEFGHIJKL
MNOPQRSTUVWXYZABC
DEFGHIJKLMNOPQRSTUV
WXYZABCDEFGHIJKLMNOPQ
RSTUVWXYZABCDEFGHIJKLMN
OPQRSTUVWXYZABCDEFGHIJKLM
NOPQRSTUVWXYZABCDEFGHIJKLMN
Note: Inside second nested while loop we are checking for the condition – if alphabet is equal to 90(ASCII value of Z), and then reinitializing it to 64(but ASCII value of A is 65), that is because after the if condition is executed we have alphabet++; statement which increments the value of variable alphabet from 64 to 65(which is the ASCII value of A).
Alphabets and ASCII Value
We can even write the code in if else block as shown below:
i = 0;
while(i < (2*count-1))
{
printf("%c", alphabet);
if(alphabet == 90)
{
alphabet = 65;
}
else
{
alphabet++;
}
i++;
}
In this case we assign the value of variable alphabet to 65(ASCII value of A) once it has reached a value of 90(ASCII value of Z).
For list of all c programming interviews / viva question and answers visit: C Programming Interview / Viva Q&A List
For full C programming language free video tutorial list visit:C Programming: Beginner To Advance To Expert