Today let us learn a very important lesson in any web application development i.e., setting up the routes.
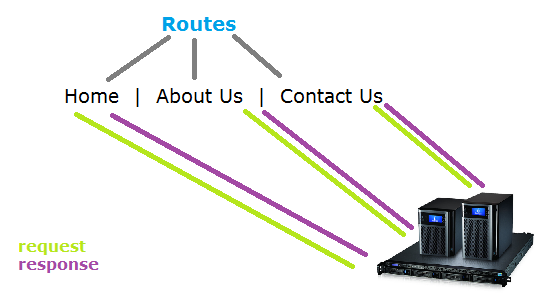
Express is an excellent web framework for Node.js
Basic Routing with Express
app.js
1
2
3
| app.get('/', function(req, res){
res.send("Hello World!");
}); |
app.get('/', function(req, res){ res.send("Hello World!");
});
This would output Hello World when users access the index or home page.
Basic Routing with Express
app.js
1
2
3
| app.get('/myPhone', function(req, res){
res.send("Sony Xperia!");
}); |
app.get('/myPhone', function(req, res){ res.send("Sony Xperia!");
});
This would output Sony Xperia! when users access the /myPhone route.
Dynamic Routing with Express
app.js
1
2
3
| app.get('/user/:username', function(req, res){
res.send(" "+req.params.username+"'s profile!");
}); |
app.get('/user/:username', function(req, res){ res.send(" "+req.params.username+"'s profile!");
});
When we request information of particular user by using his username in the URL, it fetches the username using request object and displays appropriate message.
Example: If the user requests /user/Satish it’ll output Satish’s profile!
Public Folder
If we put some files inside our public directory, it would be convenient if some middlewares fetch the files directly upon user request, instead of writing routes for all those files. Connect module which is a dependency of Express web framework takes care of this.
Middleware for public folder files
app.js
1
2
3
4
5
6
| var express = require('express');
var path = require('path');
var app = express();
app.use(express.static(path.join(__dirname, 'public'))); |
var express = require('express');
var path = require('path');
var app = express(); app.use(express.static(path.join(__dirname, 'public')));
This would set the public directory.
Middleware for public folder files
app.js
1
2
| app.use(app.router);
app.use(express.static(path.join(__dirname, 'public'))); |
app.use(app.router); app.use(express.static(path.join(__dirname, 'public')));
If you want your custom routes to be checked before the public folder, then you could specify it using another middleware, i.e., app.router
Note that, the ordering of Middleware is significant.
Sending HTML in Routs: Express
app.js
1
2
3
4
5
6
7
8
9
10
| app.get('/', function(req, res){
var msg = [
"<h1>I love Google..</h1>",
"<p>Because they make awesome products",
"<br />like my Nexus 7 Tablet",
"which is so amazing!"
].join("\n");
res.send(msg);
});
</p> |
app.get('/', function(req, res){ var msg = [ "<h1>I love Google..</h1>", "<p>Because they make awesome products", "<br />like my Nexus 7 Tablet", "which is so amazing!" ].join("\n"); res.send(msg);
});
</p>
This would out put with all HTML semantics on the browser.
Get, Post, Put, Delete Requests
Web browsers by default support only get and post requests. But we can override methods and make sure our Node.js application supports even the Put and Delete requests.
Post Request
HTML Form
index.html present in public directory
1
2
3
4
5
6
7
8
9
10
11
12
13
| < !DOCTYPE html>
<html>
<head>
<title>Enter your name</title>
</head>
<body>
<form action="/user" method="POST">
<label for="name">Name: </label>
<input type="text" name="name"/>
<input type="submit"/>
</form>
</body>
</html> |
< !DOCTYPE html>
<html>
<head>
<title>Enter your name</title>
</head>
<body>
<form action="/user" method="POST">
<label for="name">Name: </label> <input type="text" name="name"/> <input type="submit"/>
</form>
</body>
</html>
Here we have a form with post method and also take note of action field value.
POST Route
app.js
1
2
3
4
5
| app.use(express.bodyParser());
app.post('/user', function(req, res){
res.send("Submitted user's name is: "+req.body.name);
}); |
app.use(express.bodyParser());
app.post('/user', function(req, res){ res.send("Submitted user's name is: "+req.body.name);
});
Inorder to parse the HTML page, you’ll need bodyParser middleware. Once you have it in place you can get form field entries and use it to insert the data into database or simply display as in our case with this example.
We could similarly write code for PUT and DELETE requests.
PUT & DELETE Routes
app.js
1
2
3
4
5
6
7
8
9
10
| app.use(express.bodyParser());
app.use(express.methodOverride());
app.put('/user/:userId', function(req, res){
res.send("Editing user with userid: "+req.params.userId);
});
app.delete('/user/:userId', function(req, res){
res.send("Editing user with userid: "+req.params.userId);
}); |
app.use(express.bodyParser());
app.use(express.methodOverride());
app.put('/user/:userId', function(req, res){ res.send("Editing user with userid: "+req.params.userId);
});
app.delete('/user/:userId', function(req, res){ res.send("Editing user with userid: "+req.params.userId);
});
By getting the unique userId of the user, you could fetch the data from database and make changes and update the information using Put request. Similarly, using the unique userId of the user, you could select and delete the information about the user!
Basic Routing Using Express: Node.js
[youtube https://www.youtube.com/watch?v=zHykHw9JlhE]
Separating Route Files
As your application grows, its hard to keep the code cleaner and maintainable, so it’s always a good idea to separate these kind of information from the main application file. So we create a file called routes and include it as a local module in our main application file.
External Route File
/routes/index.js
1
2
3
4
5
6
7
| /*
* GET home page.
*/
exports.index = function(req, res){
res.send('Google Nexus 5 To Be Release Shortly ..');
}; |
/* * GET home page. */exports.index = function(req, res){ res.send('Google Nexus 5 To Be Release Shortly ..');
};
exports is a global provided by node.js
index is a name given by us; it’s a property name and we assign a function to it.
Accessing External Route File
app.js
1
2
3
| var routes = require('./routes');
app.get('/', routes.index); |
var routes = require('./routes');
app.get('/', routes.index);
This would output: Google Nexus 5 To Be Release Shortly ..