After learning basics of routing, lets look at some of the advances/complex routing techniques of Express.
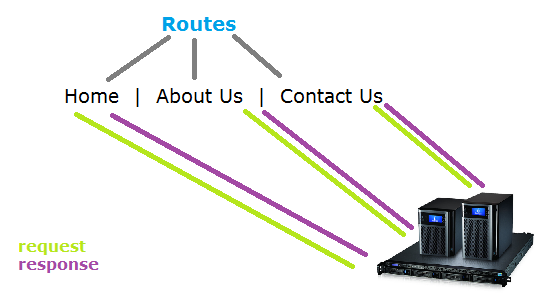
In this video tutorial, we shall teach you how to work with multiple params and also defining routes with regular expressions.
Two params in a Route: Express
app.js
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 | var companies = [ { "name": "Apple", "product": "iPhone" }, { "name": "Google", "product": "Nexus" }, { "name": "Oracle", "product": "sql" }, { "name": "Microsoft", "product": "Windows" } ]; app.get('/user/:from-:to', function(req, res){ var from = parseInt(req.params.from, 10), to = parseInt(req.params.to, 10); res.json( companies.slice(from, to+1) ); }); |
Here we have an array of objects – which in real-time application we get from a database ( Ex: MongoDB ). Now we define a route, and get two params in a single URL. By fetching and parsing those two params, we pass it to slice method of array and get array objects within the range/limit. Also note the use of response in json formatting while sending the data using response object.
Regular Expressions in Routes: Express
app.js
1 2 3 4 5 6 7 8 9 | app.get(/\/user\/(\d*)\/?(edit)?/, function(req, res){ if(req.params[0]) res.send("Viewing user id: "+req.params[0]); else if(req.params[1]) res.send("editing user with an id "+req.params[0]); else res.send("Enter User ID!!"); }); |
we enter our regular expression between two forward slashes. And to escape the forward slash present inside our regular expression, we make use of escape character i.e., a back slash. After /user/ we can have zero or more digits, after that an optional edit keyword followed by an optional trailing forward slash.
These routes match our pattern:
/user/
/user/userId/
/user/userId/edit
/user/userId/edit/
Depending on which URL the user is requesting, we could serve the purpose, using conditionals.
Advanced Routing Using Express: Node.js
[youtube https://www.youtube.com/watch?v=jYqhlVYnr30]
Note: In our example we are simply displaying the view and edit modes. But in real-time applications you could replace it with some database queries and make sure the operations makes proper sense.