Arrays is one of the most important topics in C programming language. In this video tutorial I’ll give you a brief introduction to Arrays.
Declaring Normal/regular Variable
Syntax:
Data_type variable_name;
Ex: int a;
Declaring Array Variable
Syntax:
Data_type variable_name[array_size];
Ex: int a[5];
Here array variable is a, it can hold upto 5 integer values.
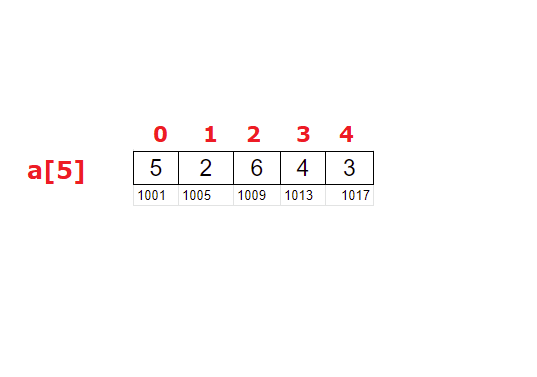
Definition of Array
An array is a collection of data items, of same data type, accessed using a common name.
Important Notes About Arrays In C
1. All the elements inside an array MUST be of same data type.
2. If you try to enter more elements than the size allocated to the array, it’ll start throwing error.
3. If you input less number of elements than the size of array, then remaining memory blocks will be filled with zeros.
4. Array variable name(without index) holds the base address or the address of first element of the array.
5. Previous address plus the size of the data type of the array gives the address of next element in the array.
Related Read:
For Loop In C Programming Language
Basics of Pointers In C Programming Language
Types of Array
There are two types of arrays in c programming:
1. One-dimensional array.
2. Multi-dimensional array.
In today’s tutorial we’ll be learning basics of one-dimensional array.
Since one-dimensional array contains some linear type of data, its also called as list or vector.
Two-dimensional arrays are often referred to as Tables or Matrix.
Video Tutorial: Introduction To Arrays: C Programming Language
[youtube https://www.youtube.com/watch?v=obyIr4dN8K0]
Source Code: Introduction To Arrays: C Programming Language
Array Read Write: integer type array
#include<stdio.h>
int main()
{
int a[5], i;
printf("Enter 5 integers\n");
for(i = 0; i < 5; i++)
{
scanf("%d", &a[i]);
}
printf("Array elements are:\n");
for(i = 0; i < 5; i++)
{
printf("%d\n", a[i]);
}
return 0;
}
Output:
Enter 5 integers
6
8
5
9
2
Array elements are:
6
8
5
9
2
Declaring and Initializing: integer type array
#include<stdio.h>
int main()
{
int a[5] = { 4, 5, 1, 9, 2 }, i;
printf("Array elements are:\n");
for(i = 0; i < 5; i++)
{
printf("%d\n", a[i]);
}
return 0;
}
Output:
Array elements are:
4
5
1
9
2
Since a[5] is of type integer, all the array elements must be integers too. we must enclose all the elements inside curly braces and each element must be separated by a comma.
Trying to insert more values than array size
#include<stdio.h>
int main()
{
int a[5] = { 4, 5, 1, 9, 2, 6 }, i;
printf("Array elements are:\n");
for(i = 0; i < 5; i++)
{
printf("%d\n", a[i]);
}
return 0;
}
Output:
warning: excess elements in array initializer.
In above source code we are trying to insert 6 integer values inside a[5], which can hold only 5 integer numbers. Hence compiler throws error and stops further compilation.
Inserting less elements/values than array size
#include<stdio.h>
int main()
{
int a[5] = { 4, 5, 1 }, i;
printf("Array elements are:\n");
for(i = 0; i < 5; i++)
{
printf("%d\n", a[i]);
}
return 0;
}
Output:
Array elements are:
4
5
1
0
0
Here array size is 5, but we’re only initializing 3 integer values. So rest of it will be filled with zeros.
To avoid conflict between number of elements and array size
#include<stdio.h>
int main()
{
int a[] = { 4, 5, 2, 6, 1, 2, 4, 5 }, i;
printf("Array elements are:\n");
for(i = 0; i < 8; i++)
{
printf("%d\n", a[i]);
}
return 0;
}
Output:
Array elements are:
4
5
2
6
1
2
4
5
Here we’re not specifying the size of array variable a. Compiler dynamically allocates size to it based on the number of integer numbers assigned to it.
Another method of assigning values to array variable
#include<stdio.h>
int main()
{
int a[3], i;
a[0] = 4;
a[1] = 5;
a[2] = 9;
printf("Array elements are:\n");
for(i = 0; i < 3; i++)
{
printf("%d\n", a[i]);
}
return 0;
}
Output:
Array elements are:
4
5
9
We could use the index and insert the value at specified position inside an array.
Note: Indexing starts from 0 in C programming language. For example, if you have an array a[5], then the elements are accessed one by one like this: a[0], a[1], a[2], a[3], a[4].
Overwriting value of elements in an array
#include<stdio.h>
int main()
{
int a[] = { 4, 5, 2, 6, 1, 2, 4, 5 }, i;
a[5] = 100;
printf("Array elements are:\n");
for(i = 0; i < 8; i++)
{
printf("%d\n", a[i]);
}
return 0;
}
Output:
Array elements are:
4
5
2
6
1
100
4
5
Here previous value of a[5], which is 2 will be replaced by 100.
Pointers and Arrays
#include<stdio.h>
int main()
{
int a[5] = { 4, 5, 2, 6, 1 };
printf("%d\n", a);
printf("%d\n", &a[0]);
return 0;
}
Output:
6356716
6356716
Here variable a will have base address or the address of first array element. In above program we’re printing the value of a and also the address where the first element of the array is stored. Both display the same address, meaning: a has base address or the address of first element in the array.
Pointers and Arrays
#include<stdio.h>
int main()
{
int a[5] = { 4, 5, 2, 6, 1 };
printf("%d\n", &a[0]);
printf("%d\n", &a[1]);
printf("%d\n", &a[3]);
printf("%d\n", &a[4]);
return 0;
}
Output:
6356716
6356720
6356728
6356732
If you observe above addresses, there is a difference of 4 between each address. That’s because each memory cell stores integer type data(in above program), which is allocated with 4 bytes of memory(it is machine dependent).
Characters and Arrays
#include<stdio.h>
int main()
{
char ch[5] = { 'A', 'P', 'P', 'L', 'E' };
int i;
for(i = 0; i < 5; i++)
printf("%c", ch[i]);
return 0;
}
Output:
APPLE
String and Arrays
#include<stdio.h>
int main()
{
char ch[5] = { 'A', 'P', 'P', 'L', 'E' };
printf("%s", ch);
return 0;
}
Output:
APPLE&
Array of characters is called as string. Observe the output of above program. It has ampersand symbol at the end. To remove this kind of random symbols we need to let the program know the end of a string.
#include<stdio.h>
int main()
{
char ch[6] = { 'A', 'P', 'P', 'L', 'E', '\0' };
printf("%s", ch);
return 0;
}
Output:
APPLE
Look at the last element in the array. Its forward slash followed by zero. That indicates end of string.
#include<stdio.h>
int main()
{
char ch[] = { 'I', 'B', 'M', '\0' };
printf("%s", ch);
return 0;
}
Output:
IBM
#include<stdio.h>
int main()
{
char ch[] = { 'I', 'B', 'M', '\0' };
printf("%d\n", &ch[0]);
printf("%d\n", &ch[1]);
printf("%d\n", &ch[2]);
return 0;
}
Output:
6356732
6356733
6356734
Character type data has 1 byte of allocated memory. Since this array stores characters in each cell, the address of consecutive element is 1 byte apart.
For list of all c programming interviews / viva question and answers visit: C Programming Interview / Viva Q&A List
For full C programming language free video tutorial list visit:C Programming: Beginner To Advance To Expert