After going through the basics of Jade, lets learn about loops and conditional statements in Jade.
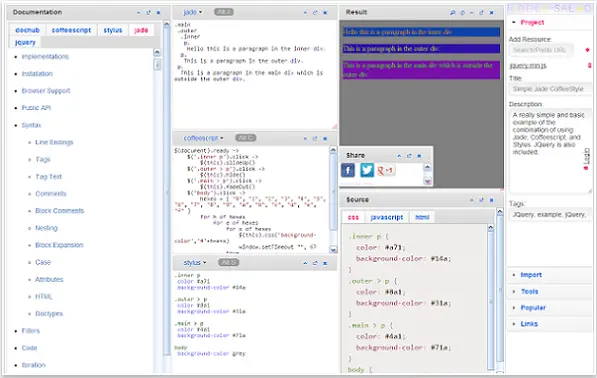
Note: Since most text editors designed for web remove the indentation(and some retain only new line characters), thus compressing the file and optimizing it for the web use. But this doesn’t serve any good for writing Jade. Because indentation is at the heart of Jade syntax!
So in this tutorial, we are using a Chrome extension called Jade Editor to illustrate loops and conditional statements. You can simply test your code in this Google Chrome extension and then copy over the code to your actual file. This would even help reduce the complicated errors in lengthy files.
each loop in Jade
arrays
1 2 3 4 5 | - names = ["Apple", "Microsoft", "Oracle"]; ul each name in names li #{name} |
output
- Apple
- Microsoft
- Oracle
– sign is optional for each loop. Here it loops through the array names and prints out each element of the array.
each loop and loop index in Jade
arrays
1 2 3 4 5 | - names = ["Apple", "Microsoft", "Oracle"]; ul each name, i in names li #{i+1}: #{name} |
output
- 1: Apple
- 2: Microsoft
- 3: Oracle
This prints out name along with the index number. Since index in JavaScript array starts with 0, we add 1 to it, to make it more sensible for non-programmers.
for loop and loop index in Jade
arrays
1 2 3 4 5 | - names = ["Apple", "Microsoft", "Oracle"]; ul for name, i in names li #{i+1}: #{name} |
output
- 1: Apple
- 2: Microsoft
- 3: Oracle
Same holds good even for for loop.
each loop in Jade
objects: Key, Value pair
1 2 3 4 5 | - obj = {cmp1: "Apple", cmp2: "IBM"}; ul each val, key in obj li #{key}: #{val} |
output
- cmp1: Apple
- cmp2: IBM
Since objects in JavaScript has {key: value} pair, we make use of it to extract and print from the object.
for loop in Jade
objects: Key, Value pair
1 2 3 4 5 | - obj = {cmp1: "Apple", cmp2: "IBM"}; ul for val, key in obj li #{key}: #{val} |
output
- cmp1: Apple
- cmp2: IBM
Same holds good to for loop too.
Conditional Statements
if, else if, else In Jade
Conditional Statements
1 2 3 4 5 6 7 8 | - var fc = 2; if( fc === 0 ) p You have no followers else if( fc === 1 ) p You have 1 follower else p You have #{fc} followers |
output
You have 2 followers
Here we use the condition to check for execution of block of code. Also note that – sign before if, else if and else keywords are optional.
Loops and Conditions In Jade: Node.js
[youtube https://www.youtube.com/watch?v=wL60uzhwwTA]
In coming video tutorials, we shall learn about Mixins.