Today lets learn about an important project which helps us use 130+ native mobile SDK features in our Ionic application through simple JavaScript interfaces – Ionic Native.
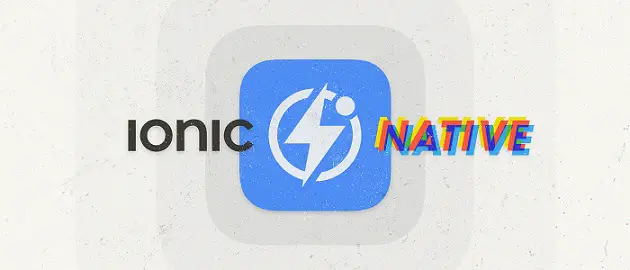
Ionic Native is a TypeScript wrapper for Cordova/PhoneGap plugins that make adding any native functionality you need to your Ionic 2 mobile app easy. Ionic Native wraps plugin callbacks in a Promise or an Observable, providing a common interface for all plugins and ensuring that native events trigger change detection in Angular 2.
Related Read:
Ionic 2 Starter Templates.
Adding AdMob In Ionic 2.
Using ‘Ionic Native’ In Ionic 2 Applications
[youtube https://www.youtube.com/watch?v=SM54loG63ks]
There are two steps required to use Ionic Native in our project
1. Install the Ionic Native package for each plugin you want to add.
2. Install the plugin using Cordova or Ionic CLI.
For example:
If we want to add AdMob plugin to our application:
Step 1: Add/ Install Specific Ionic Native package
npm install --save @ionic-native/ad-mob |
Step 2: Add / install the plugin using Cordova or Ionic CLI.
ionic plugin add cordova-plugin-admobpro |
Additional Implementation
Listing Plugin Class as Provider: src/app/app.module.ts
import { AdMob } from '@ionic-native/ad-mob'; import { NgModule, ErrorHandler } from '@angular/core'; import { IonicApp, IonicModule, IonicErrorHandler } from 'ionic-angular'; import { MyApp } from './app.component'; import { HomePage } from '../pages/home/home'; @NgModule({ declarations: [ MyApp, HomePage ], imports: [ IonicModule.forRoot(MyApp) ], bootstrap: [IonicApp], entryComponents: [ MyApp, HomePage ], providers: [ AdMob, {provide: ErrorHandler, useClass: IonicErrorHandler} ] }) export class AppModule {} |
Look at the first import statement in above code snippet. We import AdMob plugin and the way we import is specific to ad-mob and we do not import the whole ionic native script here – thus saving application load time.
Also specify the class name as provider. Because we use the services provided by this class in our application.
Working of plugin: src/app/app.component.ts
import { AdMob } from '@ionic-native/ad-mob'; import { Component } from '@angular/core'; import { Platform } from 'ionic-angular'; import { HomePage } from '../pages/home/home'; @Component({ templateUrl: 'app.html' }) export class MyApp { rootPage:any = HomePage; constructor(public admob: AdMob, platform: Platform) { platform.ready().then(() => { // Okay, so the platform is ready and our plugins are available. // Here you can do any higher level native things you might need. this.admob.createBanner({ adId: 'ca-app-pub-5732334124058455/4262868443', isTesting: false, autoShow: false }).then(() => { this.admob.showBanner(8); }); }); } } |
Again, we import AdMob native code wherever we want to implement the service of this plugin. We create instance of the class we want to implement – as constructor argument. Then inside platform.ready() method we call the methods of these classes and implement its services inside our project.
Trouble Shoot
1. If you’re getting ‘plugin not found‘ error. OR Cannot find module “@ionic-native/ “ errors, then follow below commands to fix it.
npm rebuild npm install |
2. Error Message “Provider Not Found:
Make sure to include all the specific packages inside src/app/app.module.ts file and list its class name inside providers array.