This video tutorial is an update to our previous tutorial Ionic Storage: Ionic 2.
Major Changes To Note
Previously we use to import Storage class inside src/app/app.module.ts file and we would list it as one of the providers. But now we need to import IonicStorageModule class inside src/app/app.module.ts file and list it inside imports array. That’s the only difference in implementing Ionic Storage, everything else is as described in Ionic Storage: Ionic 2 video tutorial.
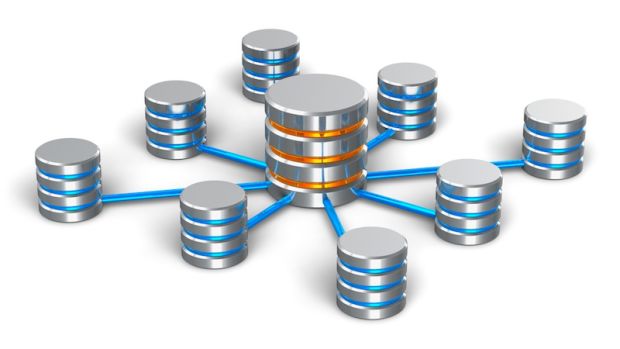
In this video tutorial only concentrate on src/app/app.module.ts file and for implementing storage set and get method refer Ionic Storage: Ionic 2 video tutorial itself.
Ionic Storage Module In Ionic 2 (Update)
[youtube https://www.youtube.com/watch?v=lKsspk5kafE]
src/app/app.module.ts
import { NgModule, ErrorHandler } from '@angular/core'; import { IonicApp, IonicModule, IonicErrorHandler } from 'ionic-angular'; import { MyApp } from './app.component'; import { HomePage } from '../pages/home/home'; import { IonicStorageModule } from '@ionic/storage'; @NgModule({ declarations: [ MyApp, HomePage ], imports: [ IonicModule.forRoot(MyApp), IonicStorageModule.forRoot() ], bootstrap: [IonicApp], entryComponents: [ MyApp, HomePage ], providers: [ {provide: ErrorHandler, useClass: IonicErrorHandler} ] }) export class AppModule {} |
Here we import IonicStorageModule class and list it inside imports array. We can also specify database name and database preference as follows:
imports: [ IonicModule.forRoot(MyApp), IonicStorageModule.forRoot({ name: '__mydbName', driverOrder: ['sqlite', 'indexeddb', 'websql'] }) ] |
Now implementing get and set methods wherever we need it. Practically it would be useful inside a data provider file, but in this tutorial am implementing inside a normal page, so that I keep the complexities out for this basic lesson.
src/pages/home/home.ts
import { Component } from '@angular/core'; import { NavController } from 'ionic-angular'; import { Storage } from '@ionic/storage'; @Component({ selector: 'page-home', templateUrl: 'home.html' }) export class HomePage { constructor(public storage: Storage, public navCtrl: NavController) { this.storage.ready().then(() => { this.storage.set('myKey', 10); }); }; getValues(){ this.storage.get('myKey').then((data) => { if(data != null) { console.log(data); } }); }; |
Here we import Storage class and create an instance of it. We check if the storage is ready. Once its ready it returns/resolves a promise. So now we can set value using this.storage.set() method. We can set an object, an array or a strong value or simply a number.
We can get the value by using its get method, which returns the value stored in the key as promise. If nothing is stored in the key, it returns null.
thank you for helping in Ionic Storage Module