Ionic 2 comes with Ionic Storage library which makes use of storage engines based on its availability and its priority. For example, in native device it uses SQLite if available, if not it’ll fall back to use localstorage or IndexedDB – again, based on its availability. If the app is being run in the web or as a Progressive Web App, Storage will attempt to use IndexedDB, WebSQL, and localstorage, in that order.
Update:
Ionic has made slight changes in how your import Ionic Storage inside app.module.ts file. You can find it at Ionic Storage Module: Ionic 2. Other than how you import Ionic Storage in app.module.ts file, everything is same as present in this(Ionic Storage: Ionic 2) video tutorial.
In this video lets learn how to store and retrieve JSON data in array format using Ionic 2 Storage with a very simple example.
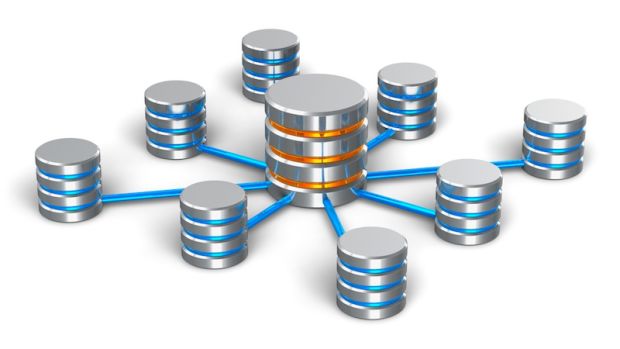
localstorage, WebSQL and IndexedDB
Since Ionic uses browser to render the application, we have access to browser based storage engines like localstorage, WebSQL and IndexedDB. But these storage engines are not reliable. If the device is running on low disk-space and the operating system decides to clear some data, then it might even clear the data stored in these storage engines.
SQLite
SQLite is query based RDBMS like Storage System for Mobile Devices. You can create, read, update and delete records just like in RDBMS. If you want to store/persist serious data locally, then you can relay on SQLite. Ionic 2 doesn’t ship with this by default – we can use cordova plugin to make use of SQLite.
cordova plugin add cordova-sqlite-storage |
Once we install this cordova plugin, Ionic 2 Storage library will priorities SQLite and make use of it instead of localstorage or IndexedDB.
Remember, Ionic Storage uses SQLite only on the native device. If you run the same application on the web maybe as a Progressive Web App, Ionic Storage will switch to use IndexedDB, WebSQL, and localstorage, in that order.
Persisting Data with Ionic Storage: Ionic 2
[youtube https://www.youtube.com/watch?v=Cnj9fQCyflY]
View Code: src/app/app.module.ts
import { NgModule, ErrorHandler } from '@angular/core'; import { IonicApp, IonicModule, IonicErrorHandler } from 'ionic-angular'; import { MyApp } from './app.component'; import { HomePage } from '../pages/home/home'; import { Storage } from '@ionic/storage'; @NgModule({ declarations: [ MyApp, HomePage ], imports: [ IonicModule.forRoot(MyApp) ], bootstrap: [IonicApp], entryComponents: [ MyApp, HomePage ], providers: [Storage, {provide: ErrorHandler, useClass: IonicErrorHandler}] }) export class AppModule {} |
Make sure to import Ionic Storage in app.module.ts file and list it as one of the providers.
Get Stored Data: src/pages/home/home.ts
constructor(public navCtrl: NavController, public storage: Storage) { this.storage.get('myStore').then((data) => { this.items = data; console.log(data); }); }; |
Make sure to import the library first inside home.ts component. Next, create a reference variable for Storage class. Now access the get method of Storage class. Using get method, get the value assocated with the given key. This returns a promise. Once the promise is resolved we get the stored data. If the key is being used for the first time and nothing has been stored yet, then it returns null.
Set Data: src/pages/home/home.ts
this.storage.set('myStore', value); |
Set the value for the given key. In my case, am using a key called myStore.
Full Free Source Code: src/pages/home/home.ts
import { Component } from '@angular/core'; import { NavController } from 'ionic-angular'; import { Storage } from '@ionic/storage'; @Component({ selector: 'page-home', templateUrl: 'home.html' }) export class HomePage { items: any; constructor(public navCtrl: NavController, public storage: Storage) { this.storage.get('myStore').then((data) => { this.items = data; console.log(data); }); }; save(val){ console.log('data added '+val); this.storage.get('myStore').then((data) => { if(data != null) { data.push(val); this.storage.set('myStore', data); } else { let array = []; array.push(val); this.storage.set('myStore', array); } }); }; } |
First we import the Storage library at the top. Next create a reference variable called storage. Inside the constructor we get / fetch the stored data and assign it to a variable called items, which we iterate through and display on the home.html view.
save() method
When the user enters some data in the input field(on the view – home.html) and submits, we check the previously stored data, if present, retrieve it, append the new data and then stored / set it back to the same key.
Full Free Source Code: src/pages/home/home.html
< ion-header> < ion-navbar> < ion-title> Ionic Blank < /ion-title> < /ion-navbar> < /ion-header> < ion-content padding> < p *ngFor="let item of items"> {{item}} < /p> < p> < ion-label fixed>Company Name< /ion-label> < ion-input type="text" name="company" [(ngModel)]="company"> < /ion-input> < button ion-button (click)="save(company);">Add< /button> < /p> < /ion-content> |
Here we iterate through the variable items and then display individual array item on the view. We also have a input field where user enters company name and once he/she hits on Add button the data gets saved in storage engine via Ionic 2 Storage.
Other Member functions of Ionic 2 Storage Class
remove(key); – Remove any value associated with this key.
clear(); – Clears the entire key value store. (Be very careful while using it.)
length(); – returns the number of keys stored.
keys(); – returns the keys in the store.
forEach(iteratorCallback) – Iterate through each key,value pair.
Hi, thanks for the video. But I just dont understend something, hope you can make me thing clear…What if this is real life app that Im using on my mobile device, would it work even when my device doesent have Internet connection, would I still get the data from SQLite? Basicaly, I dont understend Ionic 2 Storage, is it online or offline service?
@Adriano, Yes, you can still do Create, Read, Update and Delete operations when you’re offline. Because the data is being stored on the mobile device you’re using and not in the cloud..