Today lets look at editing and updating user entered data via Node.js application.
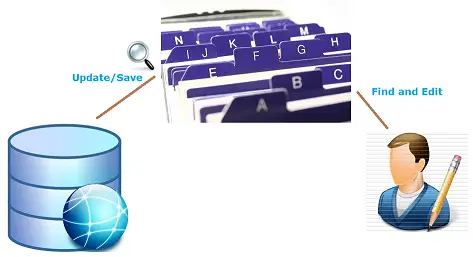
Related Read:
Connecting To MongoDB Using Mongoose: Node.js
Save data To MongoDB: Node.js
Fetch Data From MongoDB: Node.js
Fetch Individual User Data From MongoDB: Node.js
..please go through these 4 tutorials before proceeding further. It’ll take less than 20 min
link to individual profile page
view/index.jade
1
2
3
4
| ul
each user in users
li
a(href='/user/#{user._id}') #{user.name} |
ul
each user in users li a(href='/user/#{user._id}') #{user.name}
This displays list of all the users with their name, hyper-linked with their email id(present inside _id).
Adding Edit link
view/show.jade
1
2
3
4
5
6
7
8
| <h1>#{user.name}</h1>
ul
li Age: #{user.age}
li Email: #{user._id}
ul
li
a(href="/user/#{user._id}/edit") Edit |
<h1>#{user.name}</h1>
ul li Age: #{user.age} li Email: #{user._id}
ul li a(href="/user/#{user._id}/edit") Edit
This adds Edit link below each individual user information.
Edit Form
view/edit-form.jade
1
2
3
4
5
6
7
8
9
| <h1>Editing #{user.name}'s profile!</h1>
form(method="POST", action="/user/#{user._id}")
input(type="hidden", name="_method", value="PUT")
p Name:
input(type="text", name="name", value="#{user.name}")
p Age:
input(type="number", name="age", value="#{user.age}")
p
input(type="submit") |
<h1>Editing #{user.name}'s profile!</h1>
form(method="POST", action="/user/#{user._id}") input(type="hidden", name="_method", value="PUT") p Name: input(type="text", name="name", value="#{user.name}") p Age: input(type="number", name="age", value="#{user.age}") p input(type="submit")
It shows a message letting know the user, which user’s information they are editing. Also it fills the previous values for name and age. You can find a hidden field with the name _method, which helps to override POST method, and facilitates PUT method.
Edit and Update Routes
app.js
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
| app.get('/user/:id/edit', function(req, res){
res.render('edit-form', {user: req.userId});
});
app.put('/user/:id', function(req, res){
user.update({_id: req.params.id},
{
name: req.body.name,
age : req.body.age
}, function(err, docs){
if(err) res.json(err);
else res.redirect('/user/'+req.params.id);
});
});
app.param('id', function(req, res, next, id){
user.findById(id, function(err, docs){
if(err) res.json(err);
else
{
req.userId = docs;
next();
}
});
}); |
app.get('/user/:id/edit', function(req, res){
res.render('edit-form', {user: req.userId});
});
app.put('/user/:id', function(req, res){
user.update({_id: req.params.id}, { name: req.body.name, age : req.body.age }, function(err, docs){ if(err) res.json(err);
else res.redirect('/user/'+req.params.id); });
});
app.param('id', function(req, res, next, id){
user.findById(id, function(err, docs){
if(err) res.json(err);
else
{
req.userId = docs;
next();
}
});
});
Here id matches the param call, and using findById() method of mongoose, it fetches all the users present in the database. Once we call next(), it passes the control to next level. At /user/:id/edit route, we render edit-form.jade file and pass it with user information, to be filled inside the form input fields.
Once the user makes changes to the values and submits the form, all the values are passed to /user/:id route. In /user/:id route, we call update() method and specify which user information has to be updated in its first parameter, in second parameter we specify all the fields to be updated, and the third parameter is a callback method and it only takes error object as argument.
Using findByIdAndUpdate() method
app.js
1
2
3
4
5
6
7
8
9
10
11
12
13
14
| app.put('/user/:id', function(req, res){
user.findByIdAndUpdate({_id: req.params.id},
{
name: req.body.name,
age : req.body.age
}, function(err, docs){
if(err) res.json(err);
else
{
console.log(docs);
res.redirect('/user/'+req.params.id);
}
});
}); |
app.put('/user/:id', function(req, res){
user.findByIdAndUpdate({_id: req.params.id}, { name: req.body.name, age : req.body.age }, function(err, docs){ if(err) res.json(err);
else
{ console.log(docs); res.redirect('/user/'+req.params.id); } });
});
Syntax of findByIdAndUpdate() method is same as that of update(), only difference is, findByIdAndUpdate() returns the result object as well as error object to the callback method. We can make use of result object to our advantage – in our above example, I’m simply illustrating the concept by logging the result object on to the console window.
Update / Edit Document In MongoDB: Node.js
[youtube https://www.youtube.com/watch?v=wdja8tYsvK8]
Full Source Code: with findByIdAndUpdate() method
app.js
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
| var express = require('express');
var http = require('http');
var path = require('path');
var mongoose = require('mongoose');
var app = express();
// all environments
app.set('port', process.env.PORT || 3000);
app.set('views', __dirname + '/views');
app.set('view engine', 'jade');
app.use(express.bodyParser());
app.use(express.methodOverride());
app.use(app.router);
app.use(express.static(path.join(__dirname, 'public')));
mongoose.connect('mongodb://localhost/Company');
var Schema = new mongoose.Schema({
_id : String,
name: String,
age : Number
});
var user = mongoose.model('emp', Schema);
app.get('/user/:id/edit', function(req, res){
res.render('edit-form', {user: req.userId});
});
app.put('/user/:id', function(req, res){
user.findByIdAndUpdate({_id: req.params.id},
{
name: req.body.name,
age : req.body.age
}, function(err, docs){
if(err) res.json(err);
else
{
console.log(docs);
res.redirect('/user/'+req.params.id);
}
});
});
app.param('id', function(req, res, next, id){
user.findById(id, function(err, docs){
if(err) res.json(err);
else
{
req.userId = docs;
next();
}
});
});
app.get('/user/:id', function(req, res){
res.render('show', {user: req.userId});
});
app.get('/view', function(req, res){
user.find({}, function(err, docs){
if(err) res.json(err);
else res.render('index', {users: docs})
});
});
app.post('/new', function(req, res){
new user({
_id : req.body.email,
name: req.body.name,
age : req.body.age
}).save(function(err, doc){
if(err) res.json(err);
else res.redirect('/view');
});
});
var server = http.createServer(app).listen(app.get('port'), function(){
console.log('Express server listening on port ' + app.get('port'));
}); |
var express = require('express');
var http = require('http');
var path = require('path');
var mongoose = require('mongoose');
var app = express();
// all environments
app.set('port', process.env.PORT || 3000);
app.set('views', __dirname + '/views');
app.set('view engine', 'jade');
app.use(express.bodyParser());
app.use(express.methodOverride());
app.use(app.router);
app.use(express.static(path.join(__dirname, 'public')));
mongoose.connect('mongodb://localhost/Company');
var Schema = new mongoose.Schema({
_id : String,
name: String,
age : Number
});
var user = mongoose.model('emp', Schema);
app.get('/user/:id/edit', function(req, res){
res.render('edit-form', {user: req.userId});
});
app.put('/user/:id', function(req, res){
user.findByIdAndUpdate({_id: req.params.id}, { name: req.body.name, age : req.body.age }, function(err, docs){ if(err) res.json(err);
else
{ console.log(docs); res.redirect('/user/'+req.params.id); } });
});
app.param('id', function(req, res, next, id){
user.findById(id, function(err, docs){
if(err) res.json(err);
else
{
req.userId = docs;
next();
}
});
});
app.get('/user/:id', function(req, res){
res.render('show', {user: req.userId});
});
app.get('/view', function(req, res){
user.find({}, function(err, docs){
if(err) res.json(err);
else res.render('index', {users: docs})
});
});
app.post('/new', function(req, res){
new user({
_id : req.body.email,
name: req.body.name,
age : req.body.age
}).save(function(err, doc){
if(err) res.json(err);
else res.redirect('/view');
});
});
var server = http.createServer(app).listen(app.get('port'), function(){ console.log('Express server listening on port ' + app.get('port'));
});
/ for new entries
/view for viewing all the users
/user/:id to see individual user information
/user/:id/edit to edit individual user information
Note:
In real-world applications, it would make sense if only you are allowed to edit and update your data. To accomplish this – once the user logs in, store her _id value in a session variable. While the user issues an edit/update for a particular id, make sure the id she is requesting for edit/update matches with that present in the session variable!