Lets learn some of the methods and operators to work with arrays in MongoDB.
In this video tutorial, we’ll be looking at:
update()
$set
$push
$pop
$pushAll
$pull
$pullAll
$addToSet
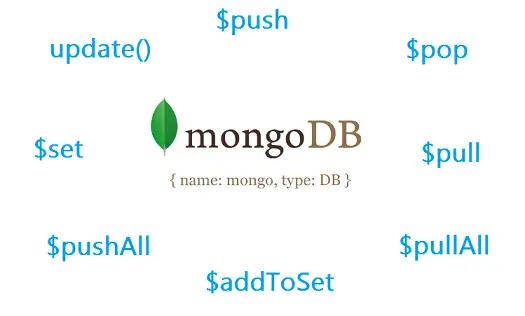
test database, names collection
1
2
3
4
5
6
7
8
9
| MongoDB shell version: 2.6.1
connecting to: test
> db.names.find()
> db.names.insert({"_id": 1, "a": [1, 2, 3, 4]});
WriteResult({ "nInserted" : 1 })
> db.names.find()
{ "_id" : 1, "a" : [ 1, 2, 3, 4 ] } |
MongoDB shell version: 2.6.1
connecting to: test
> db.names.find()
> db.names.insert({"_id": 1, "a": [1, 2, 3, 4]});
WriteResult({ "nInserted" : 1 })
> db.names.find()
{ "_id" : 1, "a" : [ 1, 2, 3, 4 ] }
We insert a document into “names” collection. We’ll be working on the array field present in the document.
Working With Arrays: MongoDB
[youtube https://www.youtube.com/watch?v=xc5TiSqvnPQ]
update() method
1
2
3
4
5
| > db.names.update({"_id": 1}, {"a": [1, 2, 3, 4, 5]});
WriteResult({ "nMatched" : 1, "nUpserted" : 0, "nModified" : 1 })
> db.names.find()
{ "_id" : 1, "a" : [ 1, 2, 3, 4, 5 ] } |
> db.names.update({"_id": 1}, {"a": [1, 2, 3, 4, 5]});
WriteResult({ "nMatched" : 1, "nUpserted" : 0, "nModified" : 1 })
> db.names.find()
{ "_id" : 1, "a" : [ 1, 2, 3, 4, 5 ] }
We can update the array by simply using update() method. But here, we need to remember all the elements of the array as well as the new element to be inserted into the array. Thus, this method is somewhat tedious.
$set operator
1
2
3
4
5
| > db.names.update({"_id": 1}, {$set: {"a.5": 6}});
WriteResult({ "nMatched" : 1, "nUpserted" : 0, "nModified" : 1 })
> db.names.find()
{ "_id" : 1, "a" : [ 1, 2, 3, 4, 5, 6 ] } |
> db.names.update({"_id": 1}, {$set: {"a.5": 6}});
WriteResult({ "nMatched" : 1, "nUpserted" : 0, "nModified" : 1 })
> db.names.find()
{ "_id" : 1, "a" : [ 1, 2, 3, 4, 5, 6 ] }
We could insert an element into the array by making use of $set operator. Here we need to know the position where the new element needs to be inserted. In mongoDB, array index starts from zero.
$push operator
1
2
3
4
5
| > db.names.update({"_id": 1}, {$push: {"a": 7}});
WriteResult({ "nMatched" : 1, "nUpserted" : 0, "nModified" : 1 })
> db.names.find()
{ "_id" : 1, "a" : [ 1, 2, 3, 4, 5, 6, 7 ] } |
> db.names.update({"_id": 1}, {$push: {"a": 7}});
WriteResult({ "nMatched" : 1, "nUpserted" : 0, "nModified" : 1 })
> db.names.find()
{ "_id" : 1, "a" : [ 1, 2, 3, 4, 5, 6, 7 ] }
using $push operator we can insert an element to the right hand side of the array. Here we simply specify the key and the value/element to be inserted.
$pop: {a: 1}
1
2
3
4
5
| > db.names.update({"_id": 1}, {$pop: {"a": 1}});
WriteResult({ "nMatched" : 1, "nUpserted" : 0, "nModified" : 1 })
> db.names.find()
{ "_id" : 1, "a" : [ 1, 2, 3, 4, 5, 6 ] } |
> db.names.update({"_id": 1}, {$pop: {"a": 1}});
WriteResult({ "nMatched" : 1, "nUpserted" : 0, "nModified" : 1 })
> db.names.find()
{ "_id" : 1, "a" : [ 1, 2, 3, 4, 5, 6 ] }
$pop operator which has 1 as value to the key, removes the right most element from the array.
$pop: {a: -1}
1
2
3
4
5
| > db.names.update({"_id": 1}, {$pop: {"a": -1}});
WriteResult({ "nMatched" : 1, "nUpserted" : 0, "nModified" : 1 })
> db.names.find()
{ "_id" : 1, "a" : [ 2, 3, 4, 5, 6 ] } |
> db.names.update({"_id": 1}, {$pop: {"a": -1}});
WriteResult({ "nMatched" : 1, "nUpserted" : 0, "nModified" : 1 })
> db.names.find()
{ "_id" : 1, "a" : [ 2, 3, 4, 5, 6 ] }
$pop operator which has -1 as value to the key, removes the left most element from the array.
$pushAll operator
1
2
3
4
5
| > db.names.update({"_id": 1}, {$pushAll: {"a": [7, 8]}});
WriteResult({ "nMatched" : 1, "nUpserted" : 0, "nModified" : 1 })
> db.names.find()
{ "_id" : 1, "a" : [ 2, 3, 4, 5, 6, 7, 8 ] } |
> db.names.update({"_id": 1}, {$pushAll: {"a": [7, 8]}});
WriteResult({ "nMatched" : 1, "nUpserted" : 0, "nModified" : 1 })
> db.names.find()
{ "_id" : 1, "a" : [ 2, 3, 4, 5, 6, 7, 8 ] }
$pushAll operator inserts all the elements(array of elements) specified, to the right hand side of the existing array.
$pull operator
1
2
3
4
5
| > db.names.update({"_id": 1}, {$pull: {"a": 3}});
WriteResult({ "nMatched" : 1, "nUpserted" : 0, "nModified" : 1 })
> db.names.find()
{ "_id" : 1, "a" : [ 2, 4, 5, 6, 7, 8 ] } |
> db.names.update({"_id": 1}, {$pull: {"a": 3}});
WriteResult({ "nMatched" : 1, "nUpserted" : 0, "nModified" : 1 })
> db.names.find()
{ "_id" : 1, "a" : [ 2, 4, 5, 6, 7, 8 ] }
$pull operator pulls or removes the specified element from the array, irrespective of its position.
$pullAll operator
1
2
3
4
5
| > db.names.update({"_id": 1}, {$pullAll: {"a": [2, 7, 8]}});
WriteResult({ "nMatched" : 1, "nUpserted" : 0, "nModified" : 1 })
> db.names.find()
{ "_id" : 1, "a" : [ 4, 5, 6 ] } |
> db.names.update({"_id": 1}, {$pullAll: {"a": [2, 7, 8]}});
WriteResult({ "nMatched" : 1, "nUpserted" : 0, "nModified" : 1 })
> db.names.find()
{ "_id" : 1, "a" : [ 4, 5, 6 ] }
$pullAll operator pulls/removes all the elements(array of elements) specified from the array, irrespective of its position.
$addToSet operator
1
2
3
4
5
| > db.names.update({"_id": 1}, {$addToSet: {"a": 3}});
WriteResult({ "nMatched" : 1, "nUpserted" : 0, "nModified" : 1 })
> db.names.find()
{ "_id" : 1, "a" : [ 4, 5, 6, 3 ] } |
> db.names.update({"_id": 1}, {$addToSet: {"a": 3}});
WriteResult({ "nMatched" : 1, "nUpserted" : 0, "nModified" : 1 })
> db.names.find()
{ "_id" : 1, "a" : [ 4, 5, 6, 3 ] }
$addToSet operator adds specified element to the array, if its not already present in the array. If the element is already present in the array, then it doesn’t add it once again.
1
2
3
4
| > db.names.update({"_id": 1}, {$addToSet: {"a": 3}});
WriteResult({ "nMatched" : 1, "nUpserted" : 0, "nModified" : 0 })
> db.names.find()
{ "_id" : 1, "a" : [ 4, 5, 6, 3 ] } |
> db.names.update({"_id": 1}, {$addToSet: {"a": 3}});
WriteResult({ "nMatched" : 1, "nUpserted" : 0, "nModified" : 0 })
> db.names.find()
{ "_id" : 1, "a" : [ 4, 5, 6, 3 ] }
If the element is already present in the array, then $addToSet doesn’t add it once again.