Today lets learn about rotate transformation. And also lets see how we can use translate transformation and rotate transformation to create simple animation.
Recommended Read:
Translate Transformation in Canvas: HTML5
Scale Transformation in Canvas: HTML5
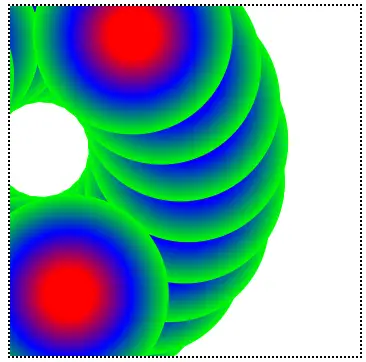
Demo
Syntax
rotate(angleInRadians);
The rotate() transformation causes the subsequent drawing operations to be rotated by a given angle. Note that the angles are in radian and not in degrees. Also the rotation takes place around the current origin and not with respect to the center of the object or the element drawn.
index.html and myStyle.css file content are same as Linear Gradients in Canvas: HTML5
JavaScript file: Rotate Transformation On a rectangle
myScript.js
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | window.onload = canvas; function canvas() { var myCanvas = document.getElementById("myCanvas"); if( myCanvas && myCanvas.getContext("2d") ) { var context = myCanvas.getContext("2d"); context.fillStyle = "blue"; context.fillRect(150, 50, 100, 50); context.rotate(0.5); context.fillRect(150, 50, 100, 50); } } |
Here we take 2 rectangles with same x and y axis and same width and height. For second rectangle we apply a rotation of 0.5 radians. With this you can see that the rotation takes place with respect to the origin of the canvas, which is (0, 0).
JavaScript file: Rotate Transformation On a Circle
myScript.js
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 | window.onload = canvas; function canvas() { var myCanvas = document.getElementById("myCanvas"); if( myCanvas && myCanvas.getContext("2d") ) { var context = myCanvas.getContext("2d"); var rdl = context.createRadialGradient(175, 175, 25, 175, 175, 100); rdl.addColorStop(0.0, "#f00"); //RED rdl.addColorStop(0.5, "#00f"); //BLUE rdl.addColorStop(1.0, "#0f0"); //Green setInterval(function(){ context.beginPath(); context.fillStyle = rdl; context.arc(175, 175, 100, 0, 2 * Math.PI); context.fill(); context.translate(175, 175); context.rotate(2); }, 50); } } |
Here we draw a radialgradient and rotate it with 2 radians with a time interval of 50 milliseconds. Before we rotate the circle we change the origin of the canvas to 175px x-axis and 175px y-axis, so that the rotation takes place with respect to the point (175, 175).
Rotate Transformation and Animation in Canvas: HTML5
[youtube https://www.youtube.com/watch?v=U3v5J-fOeV0]
Note:
We convert degree to radian using a simple mathematical formula:
Radian = (PI / 180 ) * degree;
While developing moving objects in our applications(like online games applications), we need not remember lot of locations/points on the canvas to move our element, instead, we can change the origin of the canvas using translate transformation and move the elements or rotate them!