Validating user requests is one of the key elements of a web application, and is critical for its performance.
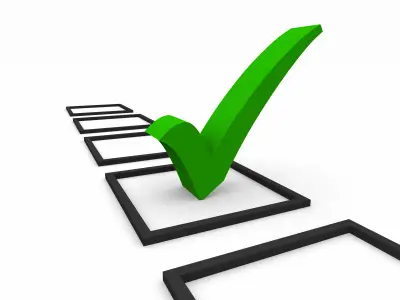
In this tutorial, we validate the user request against elements present inside an array. In real-time web applications, the request is validated against database values.
Validating User Request In Express: Node.js
app.js
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 | var names = [ "Satish", "Kiran", "Sunitha", "Jyothi" ]; app.param('username', function(req, res, next, username){ var flag = parseInt(names.indexOf(username), 10); if(flag >= 0) next(); else res.end("No Such User!"); }); app.get('/user/:username', function(req, res){ res.send("Viewing user: "+req.params.username); }); |
when the user requests for data via the route /user/someUsername we check if the user is actually present. If he is present, we’ll serve the data or else we’ll send No Such User! message to the browser.
To keep the routes clean, we shift the code to app.param First parameter indicates to which route the app.param is bound to. The callback method takes a couple of arguments – request, response, next and the username the user has requested.
we make use of indexOf() method to check if the requested username is actually present in our array. If the element is present in the array, indexOf() returns its position or else it returns -1.
If it returns 0 or any other positive value, then call next() to pass the control to the next layer of execution or else, display No Such User! and end the response.
Validating User Request: Node.js
[youtube https://www.youtube.com/watch?v=Bug_P3lxhfA]
Note: Usually if you retrieve data out of a MongoDB server, the data will be present in the form of object( {key: value} pair ).
Validating User Request In Express: Node.js
app.js
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 | var names = [ { "id" : 1, "name" : "Apple", "product": "iPhone" }, { "id" : 2, "name" : "Google", "product": "Nexus" }, { "id" : 3, "name" : "Technotip", "product": "Education" }, { "id" : 4, "name" : "Microsoft", "product": "Nokia Lumia" } ]; var flag = undefined; app.param('id', function(req, res, next, id){ for(var i = 0; i < names.length; i++ ) if(names[i].id == id) flag = "<b>Company: "+names[i].name+ "<br /><b>Product: </b>"+names[i].product; if(flag != undefined) next(); else res.end("No Such User!"); }); app.get('/user/:id', function(req, res){ res.send(flag); }); |
Here we have an array of objects. Once the user requests company information using company id(/user/:id), we check through each object’s id and if it matches we call next() or else send No Such User! to the browser.
some output
/user/0
No Such User!
/user/1
Company: Apple
Product: iPhone
/user/2
Company: Google
Product: Nexus
/user/4
Company: Microsoft
Product: Nokia Lumia
Home Work Combine today’s learning with Error handling and write complete code for user request validation as well as error handling using Error object.