In this video tutorial lets learn how we can calculate Compound Interest by making use of simple arithmetic operations.
#include < stdio.h > #include < math.h > int main() { int p, t; float r, ci; printf("Enter principal amount\n"); scanf("%d", &p); printf("Enter rate of interest\n"); scanf("%f", &r); printf("Enter time period\n"); scanf("%d", &t); ci = p * pow( (1 + r / 100), t ); printf("Compound Interest is %f\n", ci); return 0; }
Output:
Enter principal amount
1000
Enter rate of interest
8.5
Enter time period
3
Compound Interest is 1277.289185
Note: Since we’re using pow() method, we need to include math.h library file.
Scanf(): For user input
In above c program we are asking user to enter the values for variable a and b. You can know more about scanf() method/function in this video tutorial: Using Scanf in C Program
C Program to Calculate the Compound Interest
[youtube https://www.youtube.com/watch?v=DsRImGajlHw]
In this program we take input for Principal amount, rate of interest and time period from the user, and then calculate Compound Interest for those values.
Compound Interest Logic
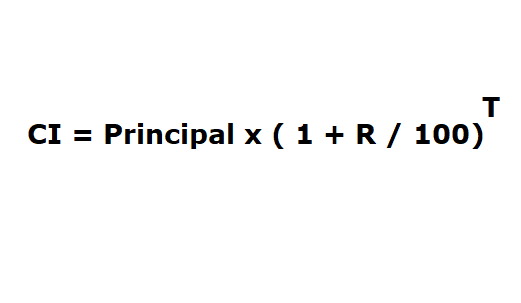
We make use of arithmetic operations available in C programming language and convert this formula to calculate Compound Interest.
Compound_Interest = Principal_amount * pow( (1 + r / 100), t );
This gives us Compound Interest and we output the result to the console.
Formula for calculating Compound Interest
p – Principal amount.
r – Rate of interest.
t – Time period.
ci – Compound Interest.
Yearly Compound Interest Formula
Compound_Interest = Principal_amount * pow( (1 + r / 100), t );
Half-Yearly Compound Interest Formula
Compound_Interest = Principal_amount * pow( (1 + (r/2) / 100), 2 * t );
Quarterly Compound Interest Formula
Compound_Interest = Principal_amount * pow( (1 + (r/4) / 100), 4 * t );
Monthly Compound Interest Formula
Compound_Interest = Principal_amount * pow( (1 + (r/12) / 100), 12 * t );
For full C programming language free video tutorial list visit:C Programming: Beginner To Advance To Expert