Today let us see REPL in Node.js
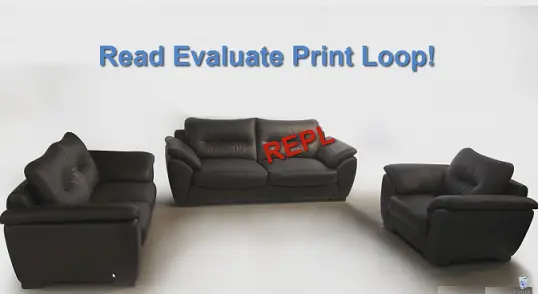
Most programming languages provide REPL facility to test and debug the code, and Node.js is no different in this approach.
Command Prompt
Console Window
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
| C:\>node
> 1 + 1
2
> var a;
undefined
> a = 10
10
> a + 5
15
> a
10
> ++a
11
> a++
11
> a
12
> "Satish "+"B"
'Satish B'
> function technotip() { return 101; }
undefined
> technotip();
101
>2 |
C:\>node
> 1 + 1
2
> var a;
undefined
> a = 10
10
> a + 5
15
> a
10
> ++a
11
> a++
11
> a
12
> "Satish "+"B"
'Satish B'
> function technotip() { return 101; }
undefined
> technotip();
101
>2
Here we can type in our valid code snippet and instantly get the results. Some of the things we can do are: arithmetic operations, string manipulations, pre and post increment decrement of numbers, method declaration and defining and calling the methods etc.
We could even require some built-in node modules and using its objects we can look at the methods and properties it provides.
Command Prompt
Console Window
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
| > var http = require("http");
undefined
> http.STATUS_CODES
{ '100': 'Continue',
'101': 'Switching Protocols',
'102': 'Processing',
'200': 'OK',
'201': 'Created',
'202': 'Accepted',
'203': 'Non-Authoritative Information',
'204': 'No Content',
'205': 'Reset Content',
'206': 'Partial Content',
'207': 'Multi-Status',
'300': 'Multiple Choices',
'301': 'Moved Permanently',
'302': 'Moved Temporarily',
'303': 'See Other',
'304': 'Not Modified',
'305': 'Use Proxy',
'307': 'Temporary Redirect',
'400': 'Bad Request',
'401': 'Unauthorized',
'402': 'Payment Required',
'403': 'Forbidden',
'404': 'Not Found',
'405': 'Method Not Allowed',
'406': 'Not Acceptable',
'407': 'Proxy Authentication Required',
'408': 'Request Time-out',
'409': 'Conflict',
'410': 'Gone',
'411': 'Length Required',
'412': 'Precondition Failed',
'413': 'Request Entity Too Large',
'414': 'Request-URI Too Large',
'415': 'Unsupported Media Type',
'416': 'Requested Range Not Satisfiable',
'417': 'Expectation Failed',
'418': 'I\'m a teapot',
'422': 'Unprocessable Entity',
'423': 'Locked',
'424': 'Failed Dependency',
'425': 'Unordered Collection',
'426': 'Upgrade Required',
'428': 'Precondition Required',
'429': 'Too Many Requests',
'431': 'Request Header Fields Too Large',
'500': 'Internal Server Error',
'501': 'Not Implemented',
'502': 'Bad Gateway',
'503': 'Service Unavailable',
'504': 'Gateway Time-out',
'505': 'HTTP Version Not Supported',
'506': 'Variant Also Negotiates',
'507': 'Insufficient Storage',
'509': 'Bandwidth Limit Exceeded',
'510': 'Not Extended',
'511': 'Network Authentication Required' } |
> var http = require("http");
undefined
> http.STATUS_CODES
{ '100': 'Continue', '101': 'Switching Protocols', '102': 'Processing', '200': 'OK', '201': 'Created', '202': 'Accepted', '203': 'Non-Authoritative Information', '204': 'No Content', '205': 'Reset Content', '206': 'Partial Content', '207': 'Multi-Status', '300': 'Multiple Choices', '301': 'Moved Permanently', '302': 'Moved Temporarily', '303': 'See Other', '304': 'Not Modified', '305': 'Use Proxy', '307': 'Temporary Redirect', '400': 'Bad Request', '401': 'Unauthorized', '402': 'Payment Required', '403': 'Forbidden', '404': 'Not Found', '405': 'Method Not Allowed', '406': 'Not Acceptable', '407': 'Proxy Authentication Required', '408': 'Request Time-out', '409': 'Conflict', '410': 'Gone', '411': 'Length Required', '412': 'Precondition Failed', '413': 'Request Entity Too Large', '414': 'Request-URI Too Large', '415': 'Unsupported Media Type', '416': 'Requested Range Not Satisfiable', '417': 'Expectation Failed', '418': 'I\'m a teapot', '422': 'Unprocessable Entity', '423': 'Locked', '424': 'Failed Dependency', '425': 'Unordered Collection', '426': 'Upgrade Required', '428': 'Precondition Required', '429': 'Too Many Requests', '431': 'Request Header Fields Too Large', '500': 'Internal Server Error', '501': 'Not Implemented', '502': 'Bad Gateway', '503': 'Service Unavailable', '504': 'Gateway Time-out', '505': 'HTTP Version Not Supported', '506': 'Variant Also Negotiates', '507': 'Insufficient Storage', '509': 'Bandwidth Limit Exceeded', '510': 'Not Extended', '511': 'Network Authentication Required' }
Here by using http object, we get all the status code supported by http module of node.js
Command Prompt
Console Window
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
| > var os = require("os");
undefined
> os
{ endianness: [Function],
hostname: [Function],
loadavg: [Function],
uptime: [Function],
freemem: [Function],
totalmem: [Function],
cpus: [Function],
type: [Function],
release: [Function],
networkInterfaces: [Function],
arch: [Function],
platform: [Function],
tmpdir: [Function],
tmpDir: [Function],
getNetworkInterfaces: [Function: deprecated],
EOL: '\r\n' }
> os.type
[Function]
> os.type();
'Windows_NT' |
> var os = require("os");
undefined
> os
{ endianness: [Function], hostname: [Function], loadavg: [Function], uptime: [Function], freemem: [Function], totalmem: [Function], cpus: [Function], type: [Function], release: [Function], networkInterfaces: [Function], arch: [Function], platform: [Function], tmpdir: [Function], tmpDir: [Function], getNetworkInterfaces: [Function: deprecated], EOL: '\r\n' }
> os.type
[Function]
> os.type();
'Windows_NT'
Here we see contents of os module. Also fetch the type of Operating System we are using, by using type() method supported by OS module.
Read Evaluate Print Loop (REPL): Node.js
[youtube https://www.youtube.com/watch?v=F2tYaJADVos]
Note: Node.js is built on the same Google’s V8 JavaScript Engine which is using by Google’s Chrome – thus providing the same speed and robustness to our node.js applications.
For quick reference: Status Code and it’s meaning
‘100’: ‘Continue’,
‘101’: ‘Switching Protocols’,
‘102’: ‘Processing’,
‘200’: ‘OK’,
‘201’: ‘Created’,
‘202’: ‘Accepted’,
‘203’: ‘Non-Authoritative Information’,
‘204’: ‘No Content’,
‘205’: ‘Reset Content’,
‘206’: ‘Partial Content’,
‘207’: ‘Multi-Status’,
‘300’: ‘Multiple Choices’,
‘301’: ‘Moved Permanently’,
‘302’: ‘Moved Temporarily’,
‘303’: ‘See Other’,
‘304’: ‘Not Modified’,
‘305’: ‘Use Proxy’,
‘307’: ‘Temporary Redirect’,
‘400’: ‘Bad Request’,
‘401’: ‘Unauthorized’,
‘402’: ‘Payment Required’,
‘403’: ‘Forbidden’,
‘404’: ‘Not Found’,
‘405’: ‘Method Not Allowed’,
‘406’: ‘Not Acceptable’,
‘407’: ‘Proxy Authentication Required’,
‘408’: ‘Request Time-out’,
‘409’: ‘Conflict’,
‘410’: ‘Gone’,
‘411’: ‘Length Required’,
‘412’: ‘Precondition Failed’,
‘413’: ‘Request Entity Too Large’,
‘414’: ‘Request-URI Too Large’,
‘415’: ‘Unsupported Media Type’,
‘416’: ‘Requested Range Not Satisfiable’,
‘417’: ‘Expectation Failed’,
‘418’: ‘I\’m a teapot’,
‘422’: ‘Unprocessable Entity’,
‘423’: ‘Locked’,
‘424’: ‘Failed Dependency’,
‘425’: ‘Unordered Collection’,
‘426’: ‘Upgrade Required’,
‘428’: ‘Precondition Required’,
‘429’: ‘Too Many Requests’,
‘431’: ‘Request Header Fields Too Large’,
‘500’: ‘Internal Server Error’,
‘501’: ‘Not Implemented’,
‘502’: ‘Bad Gateway’,
‘503’: ‘Service Unavailable’,
‘504’: ‘Gateway Time-out’,
‘505’: ‘HTTP Version Not Supported’,
‘506’: ‘Variant Also Negotiates’,
‘507’: ‘Insufficient Storage’,
‘509’: ‘Bandwidth Limit Exceeded’,
‘510’: ‘Not Extended’,
‘511’: ‘Network Authentication Required’