Lets learn how to remove documents from the collection using remove() and drop() methods.
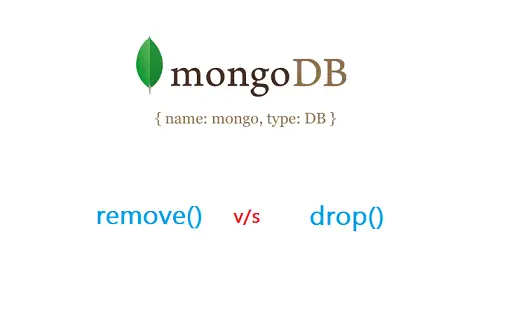
test database, names collection
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
| > db.names.find().pretty()
{
"_id" : ObjectId("53c6392a2eea8062e084cb57"),
"Company" : "Google",
"Product" : "Nexus",
"No" : 1
}
{
"_id" : ObjectId("53c639392eea8062e084cb58"),
"Company" : "Apple",
"Product" : "Mac",
"No" : 2
}
{
"_id" : ObjectId("53c63b26b003603dfdcf8c52"),
"Company" : "Xiaomi",
"Product" : "Mi3",
"No" : 3
}
{
"_id" : ObjectId("53c63bd1b003603dfdcf8c53"),
"Product" : "Smart Watch",
"No" : 4,
"Company" : "Sony"
} |
> db.names.find().pretty()
{ "_id" : ObjectId("53c6392a2eea8062e084cb57"), "Company" : "Google", "Product" : "Nexus", "No" : 1
}
{ "_id" : ObjectId("53c639392eea8062e084cb58"), "Company" : "Apple", "Product" : "Mac", "No" : 2
}
{ "_id" : ObjectId("53c63b26b003603dfdcf8c52"), "Company" : "Xiaomi", "Product" : "Mi3", "No" : 3
}
{ "_id" : ObjectId("53c63bd1b003603dfdcf8c53"), "Product" : "Smart Watch", "No" : 4, "Company" : "Sony"
}
We have 4 documents in “names” collection.
remove() method, with simple condition
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
| > db.names.remove({"No": 4});
WriteResult({ "nRemoved" : 1 })
> db.names.find().pretty()
{
"_id" : ObjectId("53c6392a2eea8062e084cb57"),
"Company" : "Google",
"Product" : "Nexus",
"No" : 1,
"IT" : "true"
}
{
"_id" : ObjectId("53c639392eea8062e084cb58"),
"Company" : "Apple",
"Product" : "Mac",
"No" : 2,
"IT" : "true"
}
{
"_id" : ObjectId("53c63b26b003603dfdcf8c52"),
"Company" : "Xiaomi",
"Product" : "Mi3",
"No" : 3,
"IT" : "true"
} |
> db.names.remove({"No": 4});
WriteResult({ "nRemoved" : 1 })
> db.names.find().pretty()
{ "_id" : ObjectId("53c6392a2eea8062e084cb57"), "Company" : "Google", "Product" : "Nexus", "No" : 1, "IT" : "true"
}
{ "_id" : ObjectId("53c639392eea8062e084cb58"), "Company" : "Apple", "Product" : "Mac", "No" : 2, "IT" : "true"
}
{ "_id" : ObjectId("53c63b26b003603dfdcf8c52"), "Company" : "Xiaomi", "Product" : "Mi3", "No" : 3, "IT" : "true"
}
Here the document with “No: 4” was removed from the collection.
Removing Documents: MongoDB
[youtube https://www.youtube.com/watch?v=so0_5Tdb_bQ]
remove() method, with comparison condition
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
| > db.names.remove({"No": {$gt: 2}});
WriteResult({ "nRemoved" : 1 })
> db.names.find().pretty()
{
"_id" : ObjectId("53c6392a2eea8062e084cb57"),
"Company" : "Google",
"Product" : "Nexus",
"No" : 1,
"IT" : "true"
}
{
"_id" : ObjectId("53c639392eea8062e084cb58"),
"Company" : "Apple",
"Product" : "Mac",
"No" : 2,
"IT" : "true"
} |
> db.names.remove({"No": {$gt: 2}});
WriteResult({ "nRemoved" : 1 })
> db.names.find().pretty()
{ "_id" : ObjectId("53c6392a2eea8062e084cb57"), "Company" : "Google", "Product" : "Nexus", "No" : 1, "IT" : "true"
}
{ "_id" : ObjectId("53c639392eea8062e084cb58"), "Company" : "Apple", "Product" : "Mac", "No" : 2, "IT" : "true"
}
Here whatever documents which has “No” greater than 2 got removed.
remove() method to remove all documents from the collection
1
2
3
4
5
6
7
8
9
10
11
| > db.names.remove({});
WriteResult({ "nRemoved" : 2 })
> db.names.find().pretty()
> show collections
names
system.indexes
> db.system.indexes.find().pretty()
{ "v" : 1, "key" : { "_id" : 1 }, "name" : "_id_", "ns" : "test.names" } |
> db.names.remove({});
WriteResult({ "nRemoved" : 2 })
> db.names.find().pretty()
> show collections
names
system.indexes
> db.system.indexes.find().pretty()
{ "v" : 1, "key" : { "_id" : 1 }, "name" : "_id_", "ns" : "test.names" }
If we pass empty argument to remove() method, it matches with all the documents present in the collection, hence removes all the documents one-by-one.
But it doesn’t remove the contents/documents/index present in “system.indexes” collection.
drop() method
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
| > db.names.find()
> db.names.insert({"Company": "Apple", "Product": "iPhone", "No": 1});
WriteResult({ "nInserted" : 1 })
> db.names.insert({"Company": "Google", "Product": "Nexus", "No": 2});
WriteResult({ "nInserted" : 1 })
> db.names.find().pretty()
{
"_id" : ObjectId("53c6c5d95879b1ff1f0b8356"),
"Company" : "Apple",
"Product" : "iPhone",
"No" : 1
}
{
"_id" : ObjectId("53c6392a2eea8062e084cb57"),
"Company" : "Google",
"Product" : "Nexus",
"No" : 1,
"IT" : "true"
}
> db.names.drop();
true
> db.names.find().pretty()
> db.system.indexes.find().pretty() |
> db.names.find()
> db.names.insert({"Company": "Apple", "Product": "iPhone", "No": 1});
WriteResult({ "nInserted" : 1 })
> db.names.insert({"Company": "Google", "Product": "Nexus", "No": 2});
WriteResult({ "nInserted" : 1 })
> db.names.find().pretty()
{ "_id" : ObjectId("53c6c5d95879b1ff1f0b8356"), "Company" : "Apple", "Product" : "iPhone", "No" : 1
}
{ "_id" : ObjectId("53c6392a2eea8062e084cb57"), "Company" : "Google", "Product" : "Nexus", "No" : 1, "IT" : "true"
}
> db.names.drop();
true
> db.names.find().pretty()
> db.system.indexes.find().pretty()
Since “names” collection was empty, we inserted 2 documents into it. Now we applied drop() method on the collection, which drops all the document present in the collections at once. It also removes the document/index/content present inside “system.indexes” collection.
Note: If you want to remove/drop all the documents present inside the collection, make use of drop() method, as it removes all the documents at once, its more efficient than remove({}) method which removes documents one by one. Use remove() method, when you want to remove one or a set of documents from the collection.