Today lets learn how to swap two integer numbers(using a temporary variable) using Macros in C.
Page Contents
Video Tutorial: Swap 2 Numbers Using Macros: C Program
[youtube https://www.youtube.com/watch?v=esQ-DmyPYYc]
Source Code: Swap 2 Numbers Using Macros: C Program
#include<stdio.h> #define SWAP(x, y, temp) temp = x; x = y; y = temp; int main() { int a, b, temp; printf("Enter 2 integer numbers\n"); scanf("%d%d", &a, &b); printf("Before swapping: a = %d and b = %d\n", a, b); SWAP(a, b, temp); printf("After swapping: a = %d and b = %d\n", a, b); return 0; }
Output:
Enter 2 integer numbers
20
50
Before swapping: a = 20 and b = 50
After swapping: a = 50 and b = 20
Logic To Swap Two Numbers
First value of a is transferred to temp;
Next value of b is transferred to a.
Next value of temp is transferred to b.
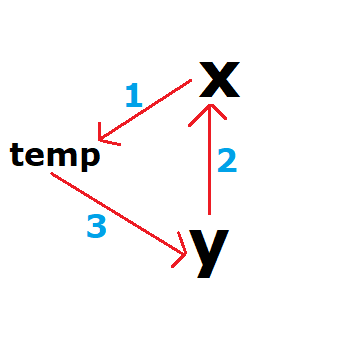
That’s how value of a and b are swapped using a temporary variable.
Note: Preprocessor replaces the macro template(SWAP(a, b, temp)) with its corresponding macro expansion(temp = x; x = y; y = temp;) before passing the source code to the compiler.
For list of all c programming interviews / viva question and answers visit: C Programming Interview / Viva Q&A List
For full C programming language free video tutorial list visit:C Programming: Beginner To Advance To Expert