We can calculate area of circle if we know the value of its radius. So using that, we shall write the logic in c program to calculate the area of a circle, without using math.h library functions.
Related Read:
Basic Arithmetic Operations In C
#include < stdio.h > int main() { float radius; const float PI = 3.14; printf("Enter the value for radius \n"); scanf("%f", &radius); printf("Area of the circle is %f\n", (PI * radius * radius)); return 0; }
Output:
Enter the value for radius
5.0
Area of the circle is 78.500003
Scanf(): For user input
In above c program we are asking user to enter the values for variable a and b. You can know more about scanf() method/function in this video tutorial: Using Scanf in C Program
Calculate Area of a Circle without using math.h library: C
[youtube https://www.youtube.com/watch?v=9-_G0N4HnLc]
Whenever we declare and initialize a constant, its a convention to make use of capital letter variable. That way we can easily spot and identify a constant in our program. And as we already know, value of constant can not be changed through out the program execution.
Ex: const float PI = 3.14;
Formula for calculating Area of a circle
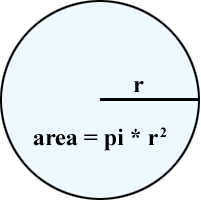
Formula for calculating Area of a circle is PI * radius * radius. We take value of radius from user and using above formula calculate area of the circle and output result to the console window.
Note that we’ve not used math.h library and any of its methods/functions in our program.
For full C programming language free video tutorial list visit:C Programming: Beginner To Advance To Expert