This video tutorial teaches about error handle of Geolocation API – of HTML5.
It is a continuation of Geolocation API – Success Handler: HTML5
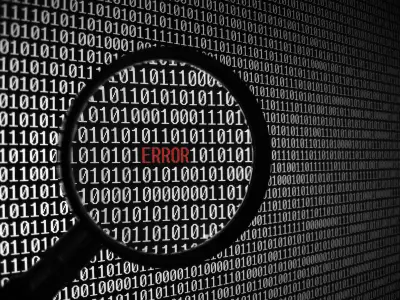
This code is helpful when geolocation API is unable to determine the users location. In this situation we, as good developers, shouldn’t leave the user keep thinking about what happened! We should gracefully inform the user about what happened in the background of our application data center.
Javascript File
myScript.js
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | function fail(error) { var errorType = { 0:"Unknown Error", 1:"Permission denied by the user", 2:"Position of the user not available", 3:"Request timed out" }; var errMsg = errorType[error.code]; if(error.code == 0 || error.code == 2){ errMsg = errMsg+" - "+error.message; } $("p").html(errMsg); } |
Geolocation API passes error object to the error handle(fail method), which contains error.code and corresponding error.message properties, using which we output the error information to the user.
Error code starts from 0 through 3, which we customize by giving our own, user understandable error messages, inside an object called errorType.
If the error code is 0 or 2, we’ll also output the original error message(sent by geolocation API) associated with the error code along with our own custom error message.
Full Free Code
Javascript File
myScript.js
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 | $(document).ready(function(){ if( navigator.geolocation ) navigator.geolocation.getCurrentPosition(success, fail); else $("p").html("HTML5 Not Supported"); }); function fail(error) { var errorType = { 0: "Unknown Error", 1: "Permission denied by the user", 2: "Position of the user not available", 3: "Request timed out" }; var errMsg = errorType[error.code]; if(error.code == 0 || error.code == 2){ errMsg = errMsg+" - "+error.message; } $("p").html(errMsg); } function success(position) { $("p").html("Latitude: "+position.coords.latitude+ "<br />Longitude: "+position.coords.longitude+ "<br />Accuracy: "+position.coords.accuracy); } |
HTML5 page(index.html) and the CSS3(myStyle.css) are same as present in our previous video tutorial.
Geolocation API – Error Handle: HTML5
[youtube https://www.youtube.com/watch?v=j_5EGTb9dhA]
We can control “request timeout” property of our application via options(3rd parameter) parameter of getCurrentPosition() method, which we’ll look in coming videos.
Next, we’ll integrate the output of geolocation API with Google Maps API and locate the actual position of the user on a MAP!