We can calculate area of circle if we know the value of its radius. So using that, we shall write the logic in c program to calculate the area of a circle, and we will us math.h library functions in this program.
Related Read:
Basic Arithmetic Operations In C
#include < stdio.h > #include < math.h > int main() { float radius; printf("Enter radius of circle\n"); scanf("%f", &radius); printf("Area of circle is %f \n", (M_PI * pow(radius, 2))); return 0; }
Output:
Enter radius of circle
2.0
Area of circle is 12.566371
Scanf(): For user input
In above c program we are asking user to enter the values for variable a and b. You can know more about scanf() method/function in this video tutorial: Using Scanf in C Program
Calculate Area of a Circle using math.h library: C
[youtube https://www.youtube.com/watch?v=eTfQ6AmwGow]
math.h library file has a constant M_PI which has the value of PI. Since it’s a constant variable we can not change its value in our program. We’re also using pow() method/function present in math.h header file.
Formula for calculating Area of a circle
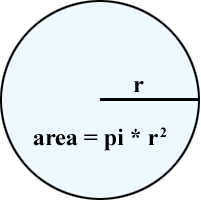
Formula for calculating Area of a circle is PI * radius * radius. We take value of radius from user and using above formula calculate area of the circle and output result to the console window. Since we’re using math.h library file we use M_PI and pow() methods for the expression.
i.e., area = M_PI * pow(radius, 2);
For full C programming language free video tutorial list visit:C Programming: Beginner To Advance To Expert