PHP & MySQL tutorial to develop signup form and store user information in a database and using signin or login form we compare for the correct username and password combination in our database. We also use session and demonstrate session_start(), session_unset(), session_destroy().
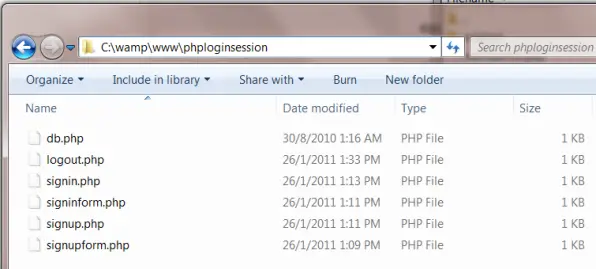
This tutorial is very important for any of your projects where you want to implement authentication: user signup and signin. So this is a crucial part of your online application. So better spend some time to understand the working. Watch the video and try to code on your own. And please make sure to contribute back to the community by commenting and sharing what you have learnt, in the comment section below.
Video Tutorial: Develop User Signup and Login forms: PHP & MySQL
[youtube https://www.youtube.com/watch?v=mn0ucCuNOTI]
In this tutorial we are using MySql database, PHP, and some HTML coding to design the forms.
Complete Source Code and Explanation:
For Database connectivity:(db.php)
<?php $conn = mysql_connect("localhost","root",""); $db = mysql_select_db("technotip",$conn); ?>
Instead of writing the database information again and again, we have moved this to one file(db.php) and call/include that file in all the scripts which needs to connect with the database.
Here localhost is the hostname. In 95% of the time the hostname will be localhost. If you are using a grid based hosting service, then it will be your grid number followed by .gridserver.com ex: xxxx.gridserver.com
We are using localhost, root is its username. We do not have any password, so we have left the next field blank.
Next line of code is to select the database present in our localhost. Here we have created a database called technotip. Below is the MySQL syntax to create the database.
Creating Database:
mysql> create database technotip; mysql> use technotip;
First line of code is to create the database, and the next line is to start making use of the created database.
Table Creation:
mysql> CREATE table phplogin( id int, username varchar(15), password varchar(20));
To keep things simple, we are creating only 3 fields in the table: id, username, password.
To Check the table description/structure:
mysql> desc phplogin;
To see the table entries:
mysql> SELECT * from phplogin;
A Form for People To SignUp(signupform.php)
<html> <form action="signup.php" method="post"> Username:<input type="text" name="n"><br /> Password:<input type="password" name="p"><br /> id :<input type="text" name="id"><br /> <input type="submit"> </form> </html>
In signupform.php we are using post method, because we will be using password and it should not be shown in the address bar of the user!
Let the name for each input field be unique, as we will be using this to receive the user input data in another file which is pointed by form action.
GET v/s POST method
The GET method produces a long string that appears in your server logs and in the browser’s address bar.
The GET method is restricted to send upto 1024 characters only.
Never use GET method if you have password or other sensitive information to be sent to the server.
GET can’t be used to send binary data, like images or word documents, to the server.
The data sent by GET method can be accessed using QUERY_STRING environment variable.
The POST method does not have any restriction on data size to be sent.
The POST method can be used to send ASCII as well as binary data.
The data sent by POST method goes through HTTP header so security depends on HTTP protocol. By using Secure HTTP you can make sure that your information is secure.
signup.php file
<?php include_once("db.php"); ?> <?php $user = $_POST['n']; $pass = $_POST['p']; $id = $_POST['id']; #$sql = "INSERT into phplogin values(".$id.",'".$user."','".$pass."')"; $sql = "INSERT into phplogin values($id,'$user','$pass')"; $qury = mysql_query($sql); # INSERT into phplogin values( # 1, # 'satish', # 'satish'); if(!$qury) { echo "Failed ".mysql_error(); echo "<br /><a href='signupform.php'>SignUp</a>"; echo "<br /><a href='signinform.php'>SignIn</a>"; } else { echo "Successful"; echo "<br /><a href='signupform.php'>SignUp</a>"; echo "<br /><a href='signinform.php'>SignIn</a>"; } ?>
Using include_once(), we are including the file db.php By including this file, we automatically get connected to the database.
We use $_POST because we have used post method in signupform.php form.
We can use either of the two MySQL query to insert the values into our table phplogin.
$sql = "INSERT into phplogin values(".$id.",'".$user."','".$pass."')"; OR $sql = "INSERT into phplogin values($id,'$user','$pass')";
Integer values need not be enclosed within single quotes, but the string variables(and values) must be enclosed within single quotation mark.
mysql_query() is used to execute the query. You can directly pass the query as parameter to this standard PHP function.
Based on the result of execution of above query we display a “Success” or “Failure” message and display some HTML links for further navigation.
Login Form: (signinform.php)
<html> <form action="signin.php" method="post"> username: <input type="text" name="name"><br /> password: <input type="password" name="pwd"><br /> <input type="submit"> </form> </html>
This is same as signupform.php form, with minor modification to the input field. And the form action is pointing to signin.php file. Here also we are using post method.
signin.php
<?php include_once("db.php"); session_start(); ?> <?php $uname = $_POST['name']; $pass = $_POST['pwd']; $sql = "SELECT count(*) FROM phplogin WHERE( username='".$uname."' and password='".$pass."')"; # SELECT count(*) FROM phplogin WHERE( # username='$uname' and password='$pass'); $qury = mysql_query($sql); $result = mysql_fetch_array($qury); if($result[0]>0) { echo "Successful Login!"; $_SESSION['userName'] = $uname; echo "<br />Welcome ".$_SESSION['userName']."!"; echo "<br /><a href='signupform.php'>SignUp</a>"; echo "<br /><a href='signinform.php'>SignIn</a>"; echo "<br /><a href='logout.php'>LogOut</a>"; } else { echo "Login Failed"; echo "<br /><a href='signupform.php'>SignUp</a>"; echo "<br /><a href='signinform.php'>SignIn</a>"; } ?>
First we include the db.php inorder to connect to the database, so that we can compare the user entered credentials with the actual username and password present in our database.
Here we are also starting the session. session_start() is the start call for the session and is a mandatory step, if you want to use session in any of your PHP script.
$sql = "SELECT count(*) FROM phplogin WHERE( username='".$uname."' and password='".$pass."')";
Using above MySQL query, we pass the user submitted username and password to our table and check if there is any presence of such combination of username and password. If the result is 1, then the username and password combination is present. If it returns 0, then there are no such a combination of username and password in the database: that means, login failed.
So, according to the result obtained we display the message and further give some links for navigation purpose.
If the login is successful, then we create a session variable with name userName and then assign the username to it.
$_SESSION['userName'] = $uname; echo "Welcome ".$_SESSION['userName']."!";
and we display Welcome userName! message, which looks like a customized welcome for each person who logs in successfully.
Once the user is logged in successfully, we provide a link to log out. Which is explained after the below snippet of code.
Logout (logout.php)
<?php session_start(); # Starts the session session_unset(); #removes all the variables in the session session_destroy(); #destroys the session if(!$_SESSION['userName']) echo "Successfully logged out!<br />"; else echo "Error Occured!!<br />"; ?>
Above code is to illustrate a simple way of working of session.
session_start(); # To Start the session
session_unset(); # Unsets/Removes all the variables in the session
session_destroy(); # Destroys the session
To demonstrate whether the logout process has removed/destroyed the set session variable, we have used the if statement, where in we check if the session variable is set or not. If still set, then the logout process isn’t working. If the session variable is destroyed/unset, then the logout successful message is displayed.
if(!$_SESSION['userName']) echo "Successfully logged out! "; else echo "Error Occurred!! ";
In above tutorial we have taken much time to link to signup and signin forms, this may look trivial; but in practice these are very important for better user experience and usability factor. So make sure you provide options for your users so that they can do something when they are landed on a page. If the page is empty and there are no links to navigate, then the user may get puzzled! Instead, if you have links to the profile and a log out link, then the user can choose, where to go next.
Please share the above video tutorial with your friends on Facebook, Twitter etc, and subscribe to our blog and YouTube channel. All the best for your application development. We are excited and eager to hear about your application development in the comment section.
HI Satish, getting error no database selected…please help me out
Check with db.php file as posted in above article. Check for proper username and password and the database name according to your database configuration.
And do not forget the command: use database_name; once you create database.
Hi satish , getting this error now
Failed Access denied for user ‘ODBC’@’localhost’ (using password: NO) on the browser , not in the cmd.
Have you created a username called ODBC ?
Check with your username and password.
By default, phpmyadmin will have username as root with not password to it, which you can leave as blank while configuring your db.php file.
hey thank Mr satish appreciate work
Great tutorial!
Question though, how would i remove the ‘id’ portion of this code with out messing it up. I’ve setup the id as an auto increments in my database so that as users register to the site an ID is automatically assigned rather then typing in one.
Thanks in advance if your able to help!
@Warkill, Simply remove the input tag associated with the id – with that you can safely remove $_POST[‘id’] and also the id field value in insert query. This would work. In the background, whenever user enters a value(registers in your case), MySQL engine assigns id to it. Hope this helps.
It did thanks :)
I managed to get it working before you got back with me but that’s what I managed to do to get it working finally.
Again this tutorial was awesome, been looking for something that works this well, that isn’t overly explained causing my mind to melt down lol.
@Warkill, Nice to know that you could set it right on your own. That is how we learn to be a better developer. Cheers!
thanks :)
Thank you sathish!
The tutorial was very useful.
Keep going!!