In this video tutorial we shall illustrate the use of comparison operators in MongoDB.
Comparison Operators
$all
$in
$nin – not in
$ne – not equal to
$gt – greater than
$gte – greater than or equal to
$lt – less than
$lte – less than or equal to
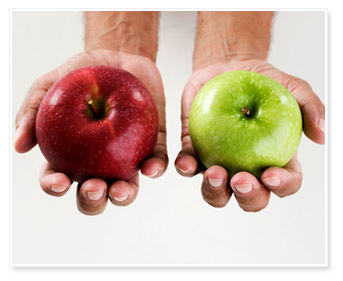
JavaScript file
load.js – in path: C:/test/load.js
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
| db.person.insert({
name : 'Satish',
age : 25,
skills : ['nodejs', 'mongoDB', 'HTML5']
});
db.person.insert({
name : 'Kiran',
age : 27,
skills : ['PHP', 'mySQL', 'HTML5']
});
db.person.insert({
name : 'Sunitha',
age : 24,
skills : ['html', 'ASP']
});
db.person.insert({
name : 'Jyothi',
age : 23,
skills : ['html', 'ASP']
});
db.person.insert({
name : 'Varsha',
age : 30,
skills : ['.NET', 'Java']
});
db.person.insert({
name : 'Amogh',
age : 29,
skills : ['C#', 'ASP']
}); |
db.person.insert({
name : 'Satish',
age : 25,
skills : ['nodejs', 'mongoDB', 'HTML5']
});
db.person.insert({
name : 'Kiran',
age : 27,
skills : ['PHP', 'mySQL', 'HTML5']
});
db.person.insert({
name : 'Sunitha',
age : 24,
skills : ['html', 'ASP']
});
db.person.insert({
name : 'Jyothi',
age : 23,
skills : ['html', 'ASP']
});
db.person.insert({
name : 'Varsha',
age : 30,
skills : ['.NET', 'Java']
});
db.person.insert({
name : 'Amogh',
age : 29,
skills : ['C#', 'ASP']
});
This JavaScript file contains some simple data, to be inserted into MongoDB server.
It contains, persons name, age and skills(in array form)
person is the collection name, we’re creating.
From our previous day video tutorial we already know, how to, Load Data From External JavaScript File: MongoDB.
load script to new database
1
2
3
4
5
6
7
8
9
| C:\mongodb>cd bin
C:\mongodb\bin>mongo 127.0.0.1/satish C:/temp/load.js
MongoDB shell version: 2.4.3
connecting to: 127.0.0.1/satish
C:\mongodb\bin>mongo
MongoDB shell version: 2.4.3
connecting to: test |
C:\mongodb>cd bin
C:\mongodb\bin>mongo 127.0.0.1/satish C:/temp/load.js
MongoDB shell version: 2.4.3
connecting to: 127.0.0.1/satish
C:\mongodb\bin>mongo
MongoDB shell version: 2.4.3
connecting to: test
Once these data/documents are loaded into new database, we start operating on this data using comparison operator.
Documents In person Collection
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
| > db.person.find().forEach(printjson)
{
"_id" : ObjectId("518c76ba05ea2a1d2b3b33a0"),
"name" : "Satish",
"age" : 25,
"skills" : [
"nodejs",
"mongoDB",
"HTML5"
]
}
{
"_id" : ObjectId("518c76ba05ea2a1d2b3b33a1"),
"name" : "Kiran",
"age" : 27,
"skills" : [
"PHP",
"mySQL",
"HTML5"
]
}
{
"_id" : ObjectId("518c76ba05ea2a1d2b3b33a2"),
"name" : "Sunitha",
"age" : 24,
"skills" : [
"html",
"ASP"
]
}
{
"_id" : ObjectId("518c76ba05ea2a1d2b3b33a3"),
"name" : "Jyothi",
"age" : 23,
"skills" : [
"html",
"ASP"
]
}
{
"_id" : ObjectId("518c76ba05ea2a1d2b3b33a4"),
"name" : "Varsha",
"age" : 30,
"skills" : [
".NET",
"Java"
]
}
{
"_id" : ObjectId("518c76ba05ea2a1d2b3b33a5"),
"name" : "Amogh",
"age" : 29,
"skills" : [
"C#",
"ASP"
]
} |
> db.person.find().forEach(printjson)
{ "_id" : ObjectId("518c76ba05ea2a1d2b3b33a0"), "name" : "Satish", "age" : 25, "skills" : [ "nodejs", "mongoDB", "HTML5" ]
}
{ "_id" : ObjectId("518c76ba05ea2a1d2b3b33a1"), "name" : "Kiran", "age" : 27, "skills" : [ "PHP", "mySQL", "HTML5" ]
}
{ "_id" : ObjectId("518c76ba05ea2a1d2b3b33a2"), "name" : "Sunitha", "age" : 24, "skills" : [ "html", "ASP" ]
}
{ "_id" : ObjectId("518c76ba05ea2a1d2b3b33a3"), "name" : "Jyothi", "age" : 23, "skills" : [ "html", "ASP" ]
}
{ "_id" : ObjectId("518c76ba05ea2a1d2b3b33a4"), "name" : "Varsha", "age" : 30, "skills" : [ ".NET", "Java" ]
}
{ "_id" : ObjectId("518c76ba05ea2a1d2b3b33a5"), "name" : "Amogh", "age" : 29, "skills" : [ "C#", "ASP" ]
}
Comparison Operators: MongoDB
[youtube https://www.youtube.com/watch?v=937PSHo7F00]
Switch to new database, ‘satish’
1
2
3
4
5
6
7
| > show dbs
admin 0.203125GB
company 0.203125GB
local 0.078125GB
satish 0.203125GB
> use satish
switched to db satish |
> show dbs
admin 0.203125GB
company 0.203125GB
local 0.078125GB
satish 0.203125GB
> use satish
switched to db satish
$all operator
1
2
3
4
| > db.person.find({skills: { $all: ['html', 'css'] }}, {name: 1, _id: 0});
> db.person.find({skills: { $all: ['html', 'ASP'] }}, {name: 1, _id: 0});
{ "name" : "Sunitha" }
{ "name" : "Jyothi" } |
> db.person.find({skills: { $all: ['html', 'css'] }}, {name: 1, _id: 0});
> db.person.find({skills: { $all: ['html', 'ASP'] }}, {name: 1, _id: 0});
{ "name" : "Sunitha" }
{ "name" : "Jyothi" }
Matches arrays that contain all elements specified in the query.
$in operator
1
2
3
4
5
6
| > db.person.find({skills: { $in: ['html', 'css'] }}, {name: 1, _id: 0});
{ "name" : "Sunitha" }
{ "name" : "Jyothi" }
> db.person.find({skills: { $in: ['java', 'css'] }}, {name: 1, _id: 0});
> db.person.find({skills: { $in: ['Java', 'css'] }}, {name: 1, _id: 0});
{ "name" : "Varsha" } |
> db.person.find({skills: { $in: ['html', 'css'] }}, {name: 1, _id: 0});
{ "name" : "Sunitha" }
{ "name" : "Jyothi" }
> db.person.find({skills: { $in: ['java', 'css'] }}, {name: 1, _id: 0});
> db.person.find({skills: { $in: ['Java', 'css'] }}, {name: 1, _id: 0});
{ "name" : "Varsha" }
Matches any of the values that exist in an array specified in the query.
$nin operator: not in
1
2
3
4
5
6
7
8
9
| > db.person.find({skills: { $nin: ['Java', 'css'] }}, {name: 1, _id: 0});
{ "name" : "Satish" }
{ "name" : "Kiran" }
{ "name" : "Sunitha" }
{ "name" : "Jyothi" }
{ "name" : "Amogh" }
> db.person.find({skills: { $nin: ['Java', 'ASP'] }}, {name: 1, _id: 0});
{ "name" : "Satish" }
{ "name" : "Kiran" } |
> db.person.find({skills: { $nin: ['Java', 'css'] }}, {name: 1, _id: 0});
{ "name" : "Satish" }
{ "name" : "Kiran" }
{ "name" : "Sunitha" }
{ "name" : "Jyothi" }
{ "name" : "Amogh" }
> db.person.find({skills: { $nin: ['Java', 'ASP'] }}, {name: 1, _id: 0});
{ "name" : "Satish" }
{ "name" : "Kiran" }
Matches values that do not exist in an array specified to the query.
$gt operator
1
2
3
4
| > db.person.find({age: { $gt: 25 }}, {name: 1, _id: 0});
{ "name" : "Kiran" }
{ "name" : "Varsha" }
{ "name" : "Amogh" } |
> db.person.find({age: { $gt: 25 }}, {name: 1, _id: 0});
{ "name" : "Kiran" }
{ "name" : "Varsha" }
{ "name" : "Amogh" }
Matches values that are greater than the value specified in the query.
$gte operator
1
2
3
4
5
| > db.person.find({age: { $gte: 25 }}, {name: 1, _id: 0});
{ "name" : "Satish" }
{ "name" : "Kiran" }
{ "name" : "Varsha" }
{ "name" : "Amogh" } |
> db.person.find({age: { $gte: 25 }}, {name: 1, _id: 0});
{ "name" : "Satish" }
{ "name" : "Kiran" }
{ "name" : "Varsha" }
{ "name" : "Amogh" }
Matches values that are equal to or greater than the value specified in the query.
Combining multiple operators: $gt, $gte and $lt operator
1
2
3
4
5
6
7
8
| > db.person.find({age: { $gte: 25, $lt: 29 }}, {name: 1, _id: 0});
{ "name" : "Satish" }
{ "name" : "Kiran" }
> db.person.find({age: { $gt: 25, $lt: 29 }}, {name: 1, _id: 0});
{ "name" : "Kiran" }
> db.person.find({age: { $gt: 25, $lte: 29 }}, {name: 1, _id: 0});
{ "name" : "Kiran" }
{ "name" : "Amogh" } |
> db.person.find({age: { $gte: 25, $lt: 29 }}, {name: 1, _id: 0});
{ "name" : "Satish" }
{ "name" : "Kiran" }
> db.person.find({age: { $gt: 25, $lt: 29 }}, {name: 1, _id: 0});
{ "name" : "Kiran" }
> db.person.find({age: { $gt: 25, $lte: 29 }}, {name: 1, _id: 0});
{ "name" : "Kiran" }
{ "name" : "Amogh" }
$lt – less than – Matches vales that are less than the value specified in the query.
$lte – less than or equal to – Matches values that are less than or equal to the value specified in the query.
$ne operator
1
2
3
4
5
6
| > db.person.find({age: {$ne: 25}}, {name: 1, _id: 0});
{ "name" : "Kiran" }
{ "name" : "Sunitha" }
{ "name" : "Jyothi" }
{ "name" : "Varsha" }
{ "name" : "Amogh" } |
> db.person.find({age: {$ne: 25}}, {name: 1, _id: 0});
{ "name" : "Kiran" }
{ "name" : "Sunitha" }
{ "name" : "Jyothi" }
{ "name" : "Varsha" }
{ "name" : "Amogh" }
Matches all values that are not equal to the value specified in the query.
Note:
Operators are useful when we do not know the exact value to be extracted or to fetch a range of values.
All mongoDB operators begin with $ sign.