In this video tutorial we illustrate the bubble sort algorithm and also see how to write it in C programming, for 5 elements as well as to N elements.
In bubble sort, a[0] is compared with a[1]. a[1] with a[2], a[2] with a[3] .. so on
if a[0] is greater than a[1], the values are swapped. This logic continues till the end of the array.
At the end of each iteration, 1 element gets sorted.
If the array size is 5, then we will have 4 iterations. i.e., if size is N, then we will have N-1 iterations.
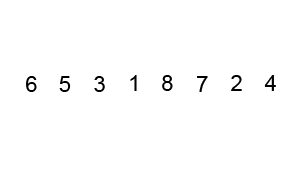
Bubble Sort: Full source code for 5 elements
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 | #include < stdio.h > #include < conio.h > void main() { int a[5] = { 15, 0, -2, 8, 3 }; int i, j, temp; clrscr(); printf("Array elements are..\n"); for( i=0; i<5; i++) printf("\n%d", a[i]); for(j=3; j>=0; j--) for(i=0; i< =j; i++) if( a[i] > a[i+1] ) { temp = a[i]; a[i] = a[i+1]; a[i+1] = temp; } printf("\nafter sorting..\n"); for(i=0; i<5; i++) printf("\n%d", a[i]); getch(); } |
Output:
Array elements are..
15
0
-2
8
3
after sorting..
-2
0
3
8
15
Video Tutorial: Bubble Sort: C
[youtube https://www.youtube.com/watch?v=Ne9QNWuGJvw]
Bubble Sort: Full source code for N elements
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 | #include < stdio.h > #include < conio.h > void main() { int a[20]; int i, j, temp, N; clrscr(); printf("\nEnter array size\n"); scanf("%d", &N); printf("Enter %d elements\n", N); for(i=0; i<n ; i++) scanf("\n%d", &a[i]); printf("Array elements are..\n"); for( i=0; i<N; i++) printf("\n%d", a[i]); for(j=N-2; j>=0; j--) for(i=0; i< =j; i++) if( a[i] > a[i+1] ) { temp = a[i]; a[i] = a[i+1]; a[i+1] = temp; } printf("\nafter sorting..\n"); for(i=0; i</n><n ; i++) printf("\n%d", a[i]); getch(); } |
Output:
Enter array size
10
Enter 10 elements
20
19
18
17
16
15
14
13
12
11
Array elements are..
20
19
18
17
16
15
14
13
12
11
after sorting..
11
12
13
14
15
16
17
18
19
20