The length and breadth / width of a rectangle are entered through the keyboard. Write a program to calculate the perimeter of the rectangle.
To get perimeter of a rectangle, we add the length and width of rectangle and then multiply it with 2. In other words, perimeter is the addition of length of all the sides of a rectangle.
Related Read:
Basic Arithmetic Operations In C
Area of Rectangle: C Program
Note: Perimeter of a Rectangle is calculated using the formula
Perimeter = 2 * (Length + Width);
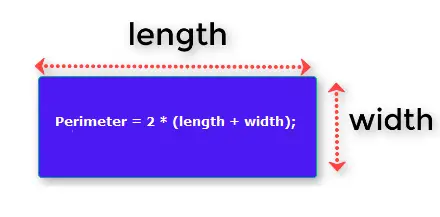
Length: is the length of the longest side of the rectangle.
width: is the length of the smallest side of the rectangle.
Find Perimeter of a Rectangle: C Program
Source Code: Find Perimeter of a Rectangle: C Program
#include < stdio.h > int main() { float length, width, perimeter; printf("Enter length of Rectangle\n"); scanf("%f", &length); printf("Enter width of Rectangle\n"); scanf("%f", &width); perimeter = 2 * (length + width); printf("Perimeter of Rectangle is %f\n", perimeter); return 0; }
Output:
Enter length of Rectangle
12
Enter width of Rectangle
6
Perimeter of Rectangle is 36.000000
For list of all c programming interviews / viva question and answers visit: C Programming Interview / Viva Q&A List
For full C programming language free video tutorial list visit:C Programming: Beginner To Advance To Expert