In today’s video tutorial, lets learn to use $exists, $type and $regex operators.
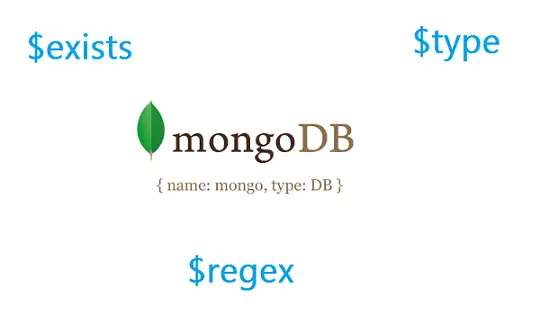
Documents in our collection
test database, names collection.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
| > use test
switched to db test
> show collections
names
system.indexes
> db.names.find().pretty()
{ "_id" : ObjectId("53be5d4604cc1cb0a7bfc3c0"), "name" : "Alia" }
{ "_id" : ObjectId("53be5d5204cc1cb0a7bfc3c1"), "name" : "Bebo" }
{ "_id" : ObjectId("53be5d5904cc1cb0a7bfc3c2"), "name" : "Chameli" }
{ "_id" : ObjectId("53be5d6104cc1cb0a7bfc3c3"), "name" : "Dev D" }
{ "_id" : ObjectId("53be5d6804cc1cb0a7bfc3c4"), "name" : "Emli" }
{ "_id" : ObjectId("53be5d8604cc1cb0a7bfc3c5"), "name" : "Farhan" }
{ "_id" : ObjectId("53be5d9204cc1cb0a7bfc3c6"), "name" : "Gangs" }
{ "_id" : ObjectId("53be5d9904cc1cb0a7bfc3c7"), "name" : "Hum" }
{ "_id" : ObjectId("53be5e3704cc1cb0a7bfc3c8"), "name" : 25 }
> db.names.insert({"name": "Satish", "age": 27});
WriteResult({ "nInserted" : 1 })
> db.names.find().pretty()
{ "_id" : ObjectId("53be5d4604cc1cb0a7bfc3c0"), "name" : "Alia" }
{ "_id" : ObjectId("53be5d5204cc1cb0a7bfc3c1"), "name" : "Bebo" }
{ "_id" : ObjectId("53be5d5904cc1cb0a7bfc3c2"), "name" : "Chameli" }
{ "_id" : ObjectId("53be5d6104cc1cb0a7bfc3c3"), "name" : "Dev D" }
{ "_id" : ObjectId("53be5d6804cc1cb0a7bfc3c4"), "name" : "Emli" }
{ "_id" : ObjectId("53be5d8604cc1cb0a7bfc3c5"), "name" : "Farhan" }
{ "_id" : ObjectId("53be5d9204cc1cb0a7bfc3c6"), "name" : "Gangs" }
{ "_id" : ObjectId("53be5d9904cc1cb0a7bfc3c7"), "name" : "Hum" }
{ "_id" : ObjectId("53be5e3704cc1cb0a7bfc3c8"), "name" : 25 }
{
"_id" : ObjectId("53beaa0f6a8a31dc255d4589"),
"name" : "Satish",
"age" : 27
} |
> use test
switched to db test
> show collections
names
system.indexes
> db.names.find().pretty()
{ "_id" : ObjectId("53be5d4604cc1cb0a7bfc3c0"), "name" : "Alia" }
{ "_id" : ObjectId("53be5d5204cc1cb0a7bfc3c1"), "name" : "Bebo" }
{ "_id" : ObjectId("53be5d5904cc1cb0a7bfc3c2"), "name" : "Chameli" }
{ "_id" : ObjectId("53be5d6104cc1cb0a7bfc3c3"), "name" : "Dev D" }
{ "_id" : ObjectId("53be5d6804cc1cb0a7bfc3c4"), "name" : "Emli" }
{ "_id" : ObjectId("53be5d8604cc1cb0a7bfc3c5"), "name" : "Farhan" }
{ "_id" : ObjectId("53be5d9204cc1cb0a7bfc3c6"), "name" : "Gangs" }
{ "_id" : ObjectId("53be5d9904cc1cb0a7bfc3c7"), "name" : "Hum" }
{ "_id" : ObjectId("53be5e3704cc1cb0a7bfc3c8"), "name" : 25 }
> db.names.insert({"name": "Satish", "age": 27});
WriteResult({ "nInserted" : 1 })
> db.names.find().pretty()
{ "_id" : ObjectId("53be5d4604cc1cb0a7bfc3c0"), "name" : "Alia" }
{ "_id" : ObjectId("53be5d5204cc1cb0a7bfc3c1"), "name" : "Bebo" }
{ "_id" : ObjectId("53be5d5904cc1cb0a7bfc3c2"), "name" : "Chameli" }
{ "_id" : ObjectId("53be5d6104cc1cb0a7bfc3c3"), "name" : "Dev D" }
{ "_id" : ObjectId("53be5d6804cc1cb0a7bfc3c4"), "name" : "Emli" }
{ "_id" : ObjectId("53be5d8604cc1cb0a7bfc3c5"), "name" : "Farhan" }
{ "_id" : ObjectId("53be5d9204cc1cb0a7bfc3c6"), "name" : "Gangs" }
{ "_id" : ObjectId("53be5d9904cc1cb0a7bfc3c7"), "name" : "Hum" }
{ "_id" : ObjectId("53be5e3704cc1cb0a7bfc3c8"), "name" : 25 }
{ "_id" : ObjectId("53beaa0f6a8a31dc255d4589"), "name" : "Satish", "age" : 27
}
Observe the documents – it has some names which are in alphabetical order. Last document has an extra field called age. And another odd entry is a document with name as 25.
$exists take 2 values, true or false
1
2
3
4
5
6
7
8
9
10
11
12
13
| > db.names.find({"age": {$exists: true}});
{ "_id" : ObjectId("53beaa0f6a8a31dc255d4589"), "name" : "Satish", "age" : 27 }
> db.names.find({"age": {$exists: false}});
{ "_id" : ObjectId("53be5d4604cc1cb0a7bfc3c0"), "name" : "Alia" }
{ "_id" : ObjectId("53be5d5204cc1cb0a7bfc3c1"), "name" : "Bebo" }
{ "_id" : ObjectId("53be5d5904cc1cb0a7bfc3c2"), "name" : "Chameli" }
{ "_id" : ObjectId("53be5d6104cc1cb0a7bfc3c3"), "name" : "Dev D" }
{ "_id" : ObjectId("53be5d6804cc1cb0a7bfc3c4"), "name" : "Emli" }
{ "_id" : ObjectId("53be5d8604cc1cb0a7bfc3c5"), "name" : "Farhan" }
{ "_id" : ObjectId("53be5d9204cc1cb0a7bfc3c6"), "name" : "Gangs" }
{ "_id" : ObjectId("53be5d9904cc1cb0a7bfc3c7"), "name" : "Hum" }
{ "_id" : ObjectId("53be5e3704cc1cb0a7bfc3c8"), "name" : 25 } |
> db.names.find({"age": {$exists: true}});
{ "_id" : ObjectId("53beaa0f6a8a31dc255d4589"), "name" : "Satish", "age" : 27 }
> db.names.find({"age": {$exists: false}});
{ "_id" : ObjectId("53be5d4604cc1cb0a7bfc3c0"), "name" : "Alia" }
{ "_id" : ObjectId("53be5d5204cc1cb0a7bfc3c1"), "name" : "Bebo" }
{ "_id" : ObjectId("53be5d5904cc1cb0a7bfc3c2"), "name" : "Chameli" }
{ "_id" : ObjectId("53be5d6104cc1cb0a7bfc3c3"), "name" : "Dev D" }
{ "_id" : ObjectId("53be5d6804cc1cb0a7bfc3c4"), "name" : "Emli" }
{ "_id" : ObjectId("53be5d8604cc1cb0a7bfc3c5"), "name" : "Farhan" }
{ "_id" : ObjectId("53be5d9204cc1cb0a7bfc3c6"), "name" : "Gangs" }
{ "_id" : ObjectId("53be5d9904cc1cb0a7bfc3c7"), "name" : "Hum" }
{ "_id" : ObjectId("53be5e3704cc1cb0a7bfc3c8"), "name" : 25 }
If $exists is true, it retrieves documents which has the specified field. If $exists is false, then it retrieves all the documents which do not have the specified field.
$exists, $type, $regex operators: MongoDB
[youtube https://www.youtube.com/watch?v=kAU4egwsBYo]
$type take numeric value(BSON specification)
1
2
3
4
5
6
7
8
9
10
11
12
13
| > db.names.find({"name": {$type: 1}});
{ "_id" : ObjectId("53be5e3704cc1cb0a7bfc3c8"), "name" : 25 }
> db.names.find({"name": {$type: 2}});
{ "_id" : ObjectId("53be5d4604cc1cb0a7bfc3c0"), "name" : "Alia" }
{ "_id" : ObjectId("53be5d5204cc1cb0a7bfc3c1"), "name" : "Bebo" }
{ "_id" : ObjectId("53be5d5904cc1cb0a7bfc3c2"), "name" : "Chameli" }
{ "_id" : ObjectId("53be5d6104cc1cb0a7bfc3c3"), "name" : "Dev D" }
{ "_id" : ObjectId("53be5d6804cc1cb0a7bfc3c4"), "name" : "Emli" }
{ "_id" : ObjectId("53be5d8604cc1cb0a7bfc3c5"), "name" : "Farhan" }
{ "_id" : ObjectId("53be5d9204cc1cb0a7bfc3c6"), "name" : "Gangs" }
{ "_id" : ObjectId("53be5d9904cc1cb0a7bfc3c7"), "name" : "Hum" }
{ "_id" : ObjectId("53beaa0f6a8a31dc255d4589"), "name" : "Satish", "age" : 27 } |
> db.names.find({"name": {$type: 1}});
{ "_id" : ObjectId("53be5e3704cc1cb0a7bfc3c8"), "name" : 25 }
> db.names.find({"name": {$type: 2}});
{ "_id" : ObjectId("53be5d4604cc1cb0a7bfc3c0"), "name" : "Alia" }
{ "_id" : ObjectId("53be5d5204cc1cb0a7bfc3c1"), "name" : "Bebo" }
{ "_id" : ObjectId("53be5d5904cc1cb0a7bfc3c2"), "name" : "Chameli" }
{ "_id" : ObjectId("53be5d6104cc1cb0a7bfc3c3"), "name" : "Dev D" }
{ "_id" : ObjectId("53be5d6804cc1cb0a7bfc3c4"), "name" : "Emli" }
{ "_id" : ObjectId("53be5d8604cc1cb0a7bfc3c5"), "name" : "Farhan" }
{ "_id" : ObjectId("53be5d9204cc1cb0a7bfc3c6"), "name" : "Gangs" }
{ "_id" : ObjectId("53be5d9904cc1cb0a7bfc3c7"), "name" : "Hum" }
{ "_id" : ObjectId("53beaa0f6a8a31dc255d4589"), "name" : "Satish", "age" : 27 }
Type | Number |
Double | 1 |
String | 2 |
Object | 3 |
Array | 4 |
Binary data | 5 |
Undefined | 6 |
Object id | 7 |
Boolean | 8 |
Date | 9 |
Null | 10 |
Regular Expression | 11 |
JavaScript | 13 |
Symbol | 14 |
JavaScript (with scope) | 15 |
32-bit integer | 16 |
Timestamp | 17 |
64-bit integer | 18 |
Min key | 255 |
Max key | 127 |
$regex (similar to perl regular expression)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
| > db.names.find({"name": {$regex: "e"}});
{ "_id" : ObjectId("53be5d5204cc1cb0a7bfc3c1"), "name" : "Bebo" }
{ "_id" : ObjectId("53be5d5904cc1cb0a7bfc3c2"), "name" : "Chameli" }
{ "_id" : ObjectId("53be5d6104cc1cb0a7bfc3c3"), "name" : "Dev D" }
> db.names.find({"name": {$regex: "^E"}});
{ "_id" : ObjectId("53be5d6804cc1cb0a7bfc3c4"), "name" : "Emli" }
> db.names.find({"name": {$regex: "h$"}});
{ "_id" : ObjectId("53beaa0f6a8a31dc255d4589"), "name" : "Satish", "age" : 27 }
> db.names.find({"name": {$regex: "^[A-Z]"}});
{ "_id" : ObjectId("53be5d4604cc1cb0a7bfc3c0"), "name" : "Alia" }
{ "_id" : ObjectId("53be5d5204cc1cb0a7bfc3c1"), "name" : "Bebo" }
{ "_id" : ObjectId("53be5d5904cc1cb0a7bfc3c2"), "name" : "Chameli" }
{ "_id" : ObjectId("53be5d6104cc1cb0a7bfc3c3"), "name" : "Dev D" }
{ "_id" : ObjectId("53be5d6804cc1cb0a7bfc3c4"), "name" : "Emli" }
{ "_id" : ObjectId("53be5d8604cc1cb0a7bfc3c5"), "name" : "Farhan" }
{ "_id" : ObjectId("53be5d9204cc1cb0a7bfc3c6"), "name" : "Gangs" }
{ "_id" : ObjectId("53be5d9904cc1cb0a7bfc3c7"), "name" : "Hum" }
{ "_id" : ObjectId("53beaa0f6a8a31dc255d4589"), "name" : "Satish", "age" : 27 }
> db.names.find({"name": {$regex: "^[A-E]"}});
{ "_id" : ObjectId("53be5d4604cc1cb0a7bfc3c0"), "name" : "Alia" }
{ "_id" : ObjectId("53be5d5204cc1cb0a7bfc3c1"), "name" : "Bebo" }
{ "_id" : ObjectId("53be5d5904cc1cb0a7bfc3c2"), "name" : "Chameli" }
{ "_id" : ObjectId("53be5d6104cc1cb0a7bfc3c3"), "name" : "Dev D" }
{ "_id" : ObjectId("53be5d6804cc1cb0a7bfc3c4"), "name" : "Emli" } |
> db.names.find({"name": {$regex: "e"}});
{ "_id" : ObjectId("53be5d5204cc1cb0a7bfc3c1"), "name" : "Bebo" }
{ "_id" : ObjectId("53be5d5904cc1cb0a7bfc3c2"), "name" : "Chameli" }
{ "_id" : ObjectId("53be5d6104cc1cb0a7bfc3c3"), "name" : "Dev D" }
> db.names.find({"name": {$regex: "^E"}});
{ "_id" : ObjectId("53be5d6804cc1cb0a7bfc3c4"), "name" : "Emli" }
> db.names.find({"name": {$regex: "h$"}});
{ "_id" : ObjectId("53beaa0f6a8a31dc255d4589"), "name" : "Satish", "age" : 27 }
> db.names.find({"name": {$regex: "^[A-Z]"}});
{ "_id" : ObjectId("53be5d4604cc1cb0a7bfc3c0"), "name" : "Alia" }
{ "_id" : ObjectId("53be5d5204cc1cb0a7bfc3c1"), "name" : "Bebo" }
{ "_id" : ObjectId("53be5d5904cc1cb0a7bfc3c2"), "name" : "Chameli" }
{ "_id" : ObjectId("53be5d6104cc1cb0a7bfc3c3"), "name" : "Dev D" }
{ "_id" : ObjectId("53be5d6804cc1cb0a7bfc3c4"), "name" : "Emli" }
{ "_id" : ObjectId("53be5d8604cc1cb0a7bfc3c5"), "name" : "Farhan" }
{ "_id" : ObjectId("53be5d9204cc1cb0a7bfc3c6"), "name" : "Gangs" }
{ "_id" : ObjectId("53be5d9904cc1cb0a7bfc3c7"), "name" : "Hum" }
{ "_id" : ObjectId("53beaa0f6a8a31dc255d4589"), "name" : "Satish", "age" : 27 }
> db.names.find({"name": {$regex: "^[A-E]"}});
{ "_id" : ObjectId("53be5d4604cc1cb0a7bfc3c0"), "name" : "Alia" }
{ "_id" : ObjectId("53be5d5204cc1cb0a7bfc3c1"), "name" : "Bebo" }
{ "_id" : ObjectId("53be5d5904cc1cb0a7bfc3c2"), "name" : "Chameli" }
{ "_id" : ObjectId("53be5d6104cc1cb0a7bfc3c3"), "name" : "Dev D" }
{ "_id" : ObjectId("53be5d6804cc1cb0a7bfc3c4"), "name" : "Emli" }
$regex: “e” retrieves all the documents in which small letter “e” is present.
$regex: “^E” retrieves all the documents which has it’s name beginning letter as “E”.
$regex: “h$” retrieves all the documents which has it’s name ending letter as “h”.
$regex: “^[A-Z]” retrieves all the documents which has it’s name starting with characters “A” to “Z”.
$regex: “^[A-E]” retrieves all the documents which has it’s name starting with characters “A” to “E”.
Note: There is more to regular expression, this video is just an introduction. We’ll cover more about regular expressions in a separate video of it’s own.