number input type indicates that the input field is used for entering numbers.
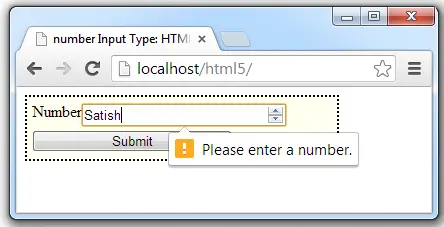
Advantages of using number input type:
1. Browsers which support HTML5, provides useful user interface enhancement by providing up and down arrow/tickers to increment and decrement the number.
2. You get a customized keyboard which is optimized for numeric inputs on mobile devices which supports number input type.
3. Using attributes like min, max and step, you can have more control. You can specify the minimum, maximum values the field takes and also you can specify the step for incrementing and decrementing the number using arrow marks.
4. Basic validation to check if the user entered numeric value or not.
HTML file
index.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | < !DOCTYPE html> <html> <head> <title>number Input Type: HTML5</title> <link href="myStyle.css" rel="stylesheet" /> </head> <body> <form> <label for="no">Number: </label> <input name="no" type="number" value="5" min="2.0" max="10.0" step="0.2" /> <input type="submit"/> </form> </body> </html> |
Here we have a number input type, with minimum allowed value of 2.0 and a maximum allowed value of 10.0 and a step of 0.2 (fraction).
CSS file associated with this is same as present at pattern and title Attribute of Form Field: HTML5
Form Input Type – number: HTML5
[youtube https://www.youtube.com/watch?v=rIiIfqS8190]
Note: Browsers which do not support number input type, simply fall back to text input type, so you can go ahead and implement number input type in your projects without worrying. It’s a good practice to even validate the code at server-side, since browsers can be easily tricked and wrong entries can be made using form elements.